WordPress is a powerful and versatile platform, but poorly optimized database queries in plugins can slow down your website and negatively impact user experience. Developers must ensure that their plugins handle database operations efficiently to maintain optimal performance and scalability. Knowing how to optimize database queries in WordPress plugins can significantly improve your site’s speed and reliability.
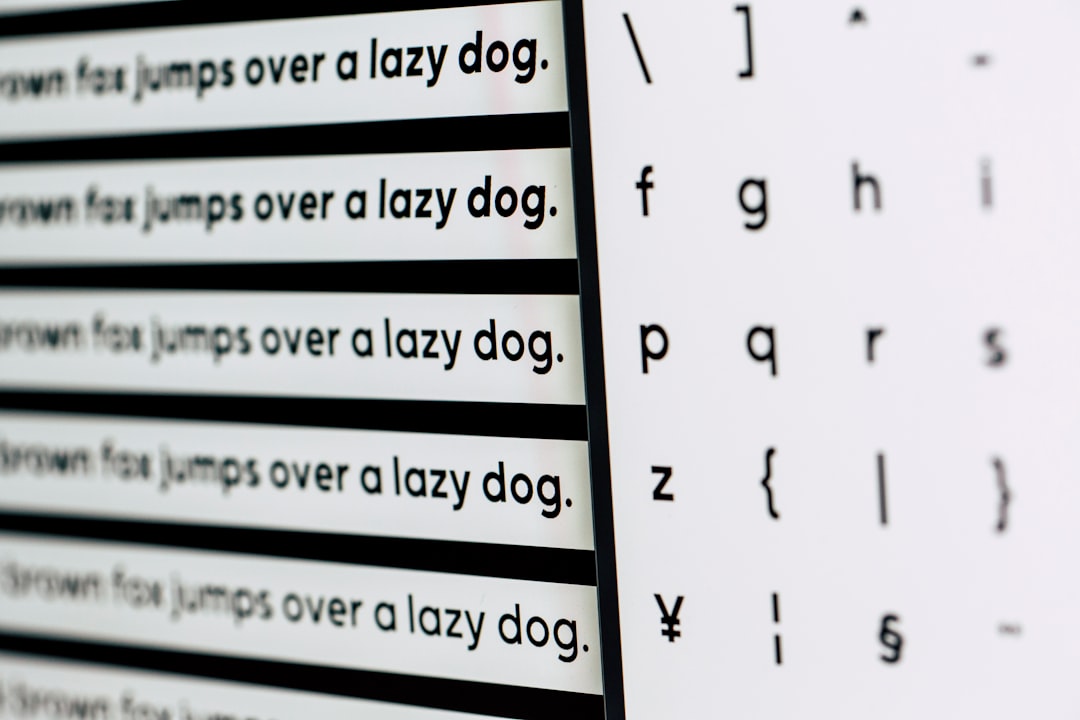
This guide covers essential techniques and best practices to write efficient queries, avoid common pitfalls, and optimize WordPress plugins for maximum performance.
Why Optimizing Database Queries Matters
The WordPress database is the backbone of your website, storing all critical data, including posts, pages, user information, and plugin settings. When plugins execute inefficient or excessive queries, they can:
- Increase server load, leading to slower page speeds.
- Cause database locks, making parts of the site unresponsive.
- Affect SEO rankings due to poor performance.
- Create scalability issues as your site grows.
By following best practices to optimize database queries in WordPress plugins, you ensure a smooth experience for your users while reducing strain on server resources.
Understanding WordPress Database Structure
Before optimizing queries, it’s essential to understand the WordPress database structure. WordPress uses a MySQL database with tables that store different types of data. Key tables include:
- wp_posts: Stores posts, pages, and custom post types.
- wp_postmeta: Contains metadata for posts.
- wp_users: Stores user data.
- wp_usermeta: Contains user-related metadata.
- wp_options: Stores site-wide settings and plugin options.
Knowing which table to query and how to join data effectively is crucial for plugin optimization.
Explore more about the database schema on the WordPress Codex.
Writing Efficient Queries with WP_Query
WP_Query is a powerful class in WordPress for querying posts and custom post types. Using it correctly can reduce unnecessary overhead in your database queries.
Use Specific Arguments
Instead of fetching all posts, narrow down your query using parameters like:
post_type
: Specify the type of content (e.g., post, page, or custom post type).posts_per_page
: Limit the number of results to prevent excessive queries.meta_query
: Filter results based on metadata conditions.
Example:
$args = array(
'post_type' => 'product',
'posts_per_page' => 10,
'meta_query' => array(
array(
'key' => 'price',
'value' => 50,
'compare' => '<=',
),
),
);
$query = new WP_Query($args);
Avoid Querying Unnecessary Fields
Fetching only the required fields using fields
can reduce query load. For example:
$args['fields'] = 'ids'; // Fetch only post IDs.
Learn more about WP_Query on the WordPress Developer Handbook.
Optimize Database Queries in WordPress Plugins with Indexing
Indexes play a critical role in speeding up database queries by allowing the database to locate rows more quickly.
Use Primary and Secondary Indexes
Ensure frequently queried columns, such as meta_key
in wp_postmeta
, are indexed. This reduces the time needed to retrieve data.
Avoid Full Table Scans
Queries without appropriate indexes force the database to scan the entire table, which is time-consuming. Use indexed columns in your WHERE
clauses.
Example:
SELECT * FROM wp_postmeta WHERE meta_key = 'featured';
Monitor Query Performance
Tools like phpMyAdmin or the MySQL EXPLAIN statement can help analyze query performance and suggest indexing improvements.
For advanced MySQL optimization tips, visit MySQL’s Performance Optimization Guide.
Caching to Improve Query Performance
Caching is one of the most effective ways to reduce database load and speed up WordPress plugins.
Use Transients for Temporary Data
Transients allow you to store cached data in the database with an expiration time. This is useful for data that doesn’t change frequently.
Example:
$cached_data = get_transient('recent_posts');
if (!$cached_data) {
$cached_data = wp_get_recent_posts(array('numberposts' => 5));
set_transient('recent_posts', $cached_data, HOUR_IN_SECONDS);
}
Leverage Object Caching
WordPress has a built-in object cache that stores query results in memory. Plugins like Redis Object Cache extend this functionality to persist cache across requests.
Avoid Redundant Queries
Store query results in a variable to prevent executing the same query multiple times.
Example:
$posts = $wpdb->get_results("SELECT ID FROM wp_posts WHERE post_status = 'publish'");
foreach ($posts as $post) {
// Process each post.
}
Learn more about caching in WordPress on WPBeginner.
Avoid Common Query Pitfalls
Even small mistakes can lead to significant performance issues. Here’s what to avoid:
Do Not Use SELECT *
Fetching all columns (SELECT *
) retrieves unnecessary data and increases query time. Instead, specify only the fields you need.
Reduce Joins
While JOIN operations are sometimes necessary, too many joins can slow down queries. Consider denormalizing data or using caching when possible.
Limit Query Results
Set limits on the number of results using LIMIT
. This ensures your queries don’t overload the database.
Example:
SELECT ID FROM wp_posts WHERE post_type = 'product' LIMIT 10;
Sanitize User Input
Always validate and sanitize input data to prevent SQL injection attacks. Use prepared statements with $wpdb
for dynamic queries.
Example:
global $wpdb;
$search_term = sanitize_text_field($_GET['search']);
$query = $wpdb->prepare("SELECT * FROM wp_posts WHERE post_title LIKE %s", '%' . $wpdb->esc_like($search_term) . '%');
$results = $wpdb->get_results($query);
Monitoring and Debugging Queries
Monitoring query performance helps you identify and resolve bottlenecks.
Use Query Monitor Plugin
Query Monitor is a powerful plugin for debugging database queries, hooks, and more.
Log Slow Queries
Enable slow query logging in MySQL to identify inefficient queries.
Example (MySQL Configuration):
slow_query_log = 1
slow_query_log_file = /var/log/mysql/slow-queries.log
long_query_time = 2
Profile Queries with EXPLAIN
Use the EXPLAIN statement to understand how MySQL executes your query and identify areas for improvement.
Example:
EXPLAIN SELECT * FROM wp_postmeta WHERE meta_key = 'color';
Conclusion
Efficient database queries are the backbone of a well-performing WordPress plugin. By learning to optimize database queries in WordPress plugins, developers can ensure their plugins are scalable, user-friendly, and resource-efficient.
Start applying these strategies today to improve your plugin’s performance and deliver a better experience for your users. For further resources, explore WordPress Plugin Developer Handbook or MySQL Documentation.