In today’s interconnected web environment, third-party APIs are essential tools for extending functionality and enhancing user experience. Integrating these APIs into your WordPress plugins can enable advanced features, such as real-time data updates, external service connections, and seamless integrations with platforms like Google, Stripe, or Mailchimp.
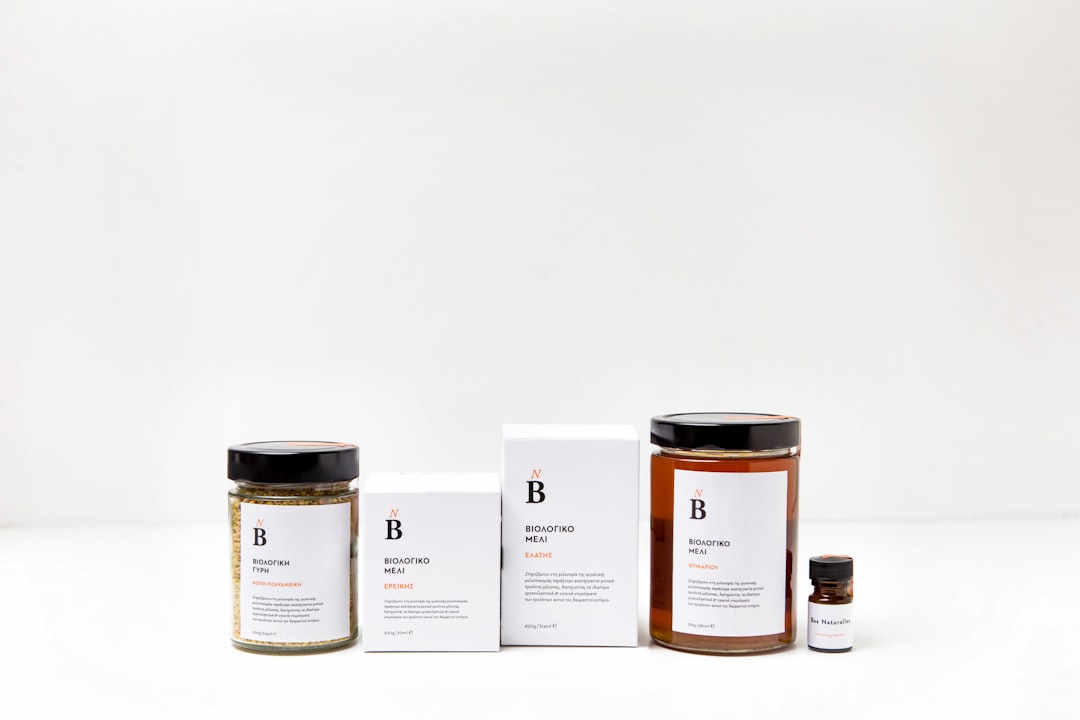
Knowing how to integrate third-party APIs effectively ensures your plugin is robust, efficient, and easy to maintain. This guide explores best practices for API integration, common challenges, and strategies to optimize performance.
Why Integrate Third-Party APIs into WordPress Plugins
Integrating third-party APIs allows developers to leverage external services and functionalities without reinventing the wheel. Here’s why APIs are a game-changer:
- Expand Plugin Functionality: APIs allow you to add complex features, such as payment gateways or social media feeds, to your plugin.
- Access Real-Time Data: APIs provide dynamic content updates, such as weather forecasts or stock prices.
- Streamline User Experience: Connecting your plugin to popular platforms enhances usability by enabling single sign-on, analytics, or data synchronization.
- Save Development Time: By utilizing pre-built APIs, you can focus on building your core plugin functionality.
For more about APIs, visit Postman’s API Learning Center.
Preparing to Integrate Third-Party APIs
Before diving into coding, it’s essential to prepare for API integration. Proper planning ensures a smooth implementation process and minimizes errors.
Understand API Documentation
Thoroughly read the API documentation provided by the third-party service. It typically includes:
- Endpoints: URLs used to interact with the API.
- Methods: HTTP methods (GET, POST, PUT, DELETE) supported by the API.
- Authentication: Details about API keys, tokens, or OAuth.
- Response Formats: Data formats like JSON or XML used by the API.
Obtain API Access Credentials
Sign up for the service and obtain API keys or tokens. These credentials are required for authentication and secure communication with the API.
Install HTTP Request Libraries
WordPress provides built-in functions for making HTTP requests, such as wp_remote_get
and wp_remote_post
. For more complex needs, libraries like Guzzle are also an option.
For additional resources, explore REST API Tutorials.
Integrate Third-Party APIs into WordPress Plugins
Adding API functionality to a WordPress plugin involves several key steps.
Register Plugin Settings for API Keys
Allow users to configure API keys directly from your plugin’s settings page.
Example:
add_action('admin_menu', 'my_plugin_menu');
function my_plugin_menu() {
add_menu_page('API Settings', 'API Settings', 'manage_options', 'api-settings', 'my_plugin_settings_page');
}
function my_plugin_settings_page() {
?>
<form method="post" action="options.php">
<?php settings_fields('api_settings'); ?>
<?php do_settings_sections('api_settings'); ?>
<label for="api_key">API Key:</label>
<input type="text" id="api_key" name="api_key" value="<?php echo esc_attr(get_option('api_key')); ?>" />
<?php submit_button(); ?>
</form>
<?php
}
add_action('admin_init', function() {
register_setting('api_settings', 'api_key');
});
Make API Requests
Use WordPress’s wp_remote_get
or wp_remote_post
functions to fetch or send data.
Example:
function fetch_api_data() {
$api_key = get_option('api_key');
$response = wp_remote_get("https://api.example.com/data?key={$api_key}");
if (is_wp_error($response)) {
return 'API request failed';
}
$data = json_decode(wp_remote_retrieve_body($response), true);
return $data;
}
Handle API Responses
Always validate and sanitize the API response before using it in your plugin.
Example:
$data = fetch_api_data();
if (isset($data['items'])) {
foreach ($data['items'] as $item) {
echo esc_html($item['name']);
}
}
Learn more about WordPress HTTP API on the WordPress Developer Handbook.
Optimize API Calls for Performance
Frequent API requests can slow down your plugin and strain the API server. Optimizing API calls ensures a better user experience and prevents service throttling.
Cache API Responses
Use the WordPress Transients API to store API responses temporarily, reducing the need for repeated requests.
Example:
function get_cached_api_data() {
$cached_data = get_transient('api_data');
if (!$cached_data) {
$cached_data = fetch_api_data();
set_transient('api_data', $cached_data, HOUR_IN_SECONDS);
}
return $cached_data;
}
Batch Requests
If the API supports it, batch multiple requests into a single call to reduce overhead.
Respect API Rate Limits
APIs often enforce rate limits. Monitor the limits and implement retry logic for failed requests.
For API optimization techniques, visit Google’s API Design Best Practices.
Use Error Handling and Logging
Errors during API integration are inevitable. Proper error handling and logging ensure smooth debugging and a better user experience.
Check for Errors in Responses
Always check for errors in API responses and handle them gracefully.
Example:
$response = fetch_api_data();
if (isset($response['error'])) {
error_log('API error: ' . $response['error']['message']);
return 'An error occurred. Please try again later.';
}
Log API Activity
Use WordPress’s built-in logging functions or plugins like WP Log Viewer to monitor API activity.
Provide User Feedback
Notify users of errors without exposing sensitive details.
Example:
if (is_wp_error($response)) {
echo 'Unable to fetch data. Please contact support.';
}
Integrate Third-Party APIs for Advanced Features
APIs can enable advanced functionality in your plugin, such as:
Payment Processing
Integrate APIs like Stripe or PayPal to enable seamless payment gateways. For example, use the Stripe PHP Library.
Social Media Integration
Fetch and display posts, tweets, or other social media content using APIs like the Facebook Graph API or Twitter API.
Data Synchronization
Synchronize your WordPress site with external CRMs or databases using APIs like Salesforce or HubSpot.
Learn more about APIs for developers on RapidAPI.
Testing and Debugging API Integrations
Thorough testing ensures your plugin functions correctly across different scenarios.
Use API Testing Tools
Tools like Postman or Insomnia help simulate API requests and understand responses.
Test with Real and Mock Data
Use real API credentials for live testing and mock data during development to avoid exceeding rate limits.
Monitor API Changes
APIs evolve, so stay updated with changelogs or announcements from the third-party provider to ensure compatibility.
Conclusion
Knowing how to integrate third-party APIs into WordPress plugins allows you to build powerful, feature-rich tools that enhance functionality and user experience. By following best practices for API requests, optimizing performance, and ensuring robust error handling, you can create plugins that are both efficient and reliable.
Start integrating APIs into your plugins today, and unlock the full potential of third-party services. For more resources, explore the WordPress Plugin Developer Handbook or Postman’s API Learning Center.