Security is paramount in WordPress plugin development, and handling user inputs safely is a critical aspect of building secure and reliable plugins. Improperly processed inputs can expose websites to vulnerabilities like cross-site scripting (XSS), SQL injection, or data corruption. Learning how to sanitize validate user inputs is essential for ensuring data integrity and protecting WordPress sites.
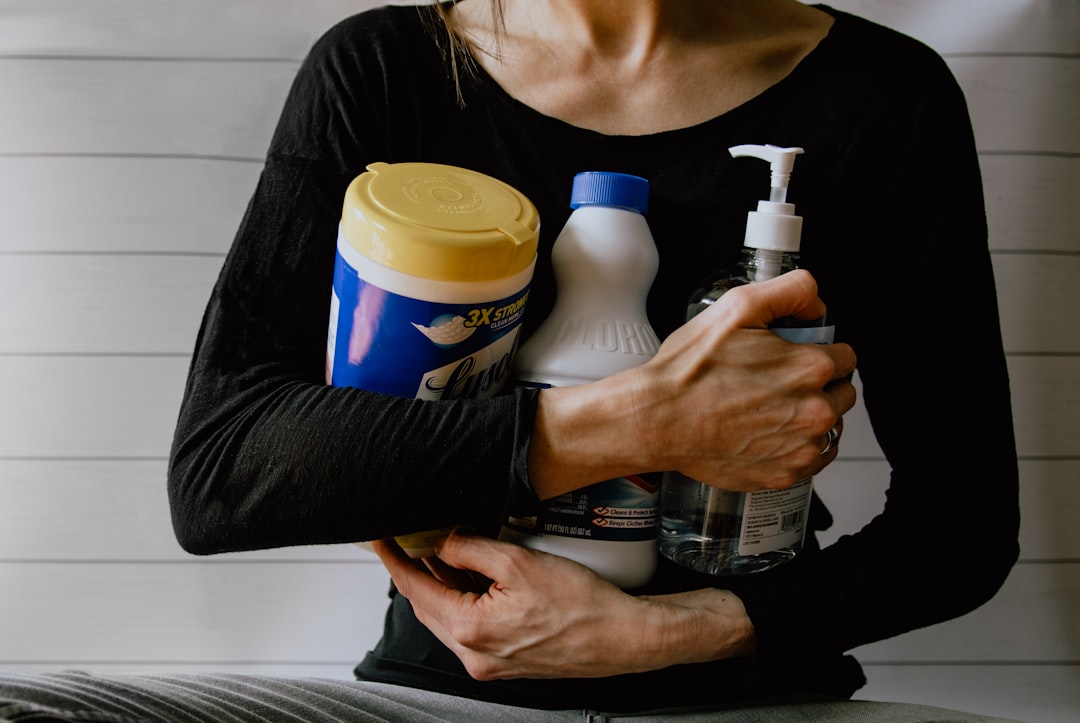
This guide provides a comprehensive overview of sanitizing and validating user inputs in plugin development, complete with examples and best practices.
Why Sanitize and Validate User Inputs?
Sanitizing and validating user inputs are two key processes that ensure data integrity and security in WordPress:
- Sanitization: Cleaning input data to remove unwanted characters or harmful code.
- Validation: Ensuring input data matches a specific format or criteria.
By combining these processes, developers can prevent vulnerabilities and maintain a secure environment.
For more on WordPress security best practices, visit WordPress Security.
Understanding Common Vulnerabilities
Cross-Site Scripting (XSS)
XSS attacks occur when malicious scripts are injected into web pages. Unsanitized user inputs can allow attackers to execute harmful scripts in the browser.
SQL Injection
SQL injection involves injecting malicious SQL queries into database operations. This can compromise sensitive data or corrupt databases.
Data Corruption
Improper handling of user inputs can result in invalid or corrupted data being stored in the database.
The Difference Between Sanitization and Validation
Sanitization
Sanitization ensures data is clean and safe to use, often by removing or encoding unwanted characters.
Example: Sanitizing a text input:
$sanitized_text = sanitize_text_field( $_POST['user_input'] );
Validation
Validation checks that input data meets specific criteria or formats.
Example: Validating an email address:
if ( is_email( $_POST['user_email'] ) ) {
$validated_email = $_POST['user_email'];
} else {
// Handle invalid email
}
WordPress Functions for Sanitization
WordPress provides built-in functions for sanitizing various types of data. Here are some commonly used functions:
sanitize_text_field()
Cleans text inputs by removing invalid characters.
$user_name = sanitize_text_field( $_POST['user_name'] );
sanitize_email()
Validates and sanitizes email addresses.
$user_email = sanitize_email( $_POST['user_email'] );
esc_url_raw()
Cleans URL inputs to ensure they are valid.
$website_url = esc_url_raw( $_POST['website_url'] );
sanitize_key()
Cleans alphanumeric keys, often used for database fields or array keys.
$option_key = sanitize_key( $_POST['option_key'] );
For a complete list of sanitization functions, visit WordPress Codex on Data Validation.
WordPress Functions for Validation
WordPress also provides functions to validate input data:
is_email()
Checks if a given string is a valid email address.
if ( is_email( $email ) ) {
// Proceed
}
validate_file()
Ensures file paths are valid and secure.
if ( validate_file( $file_path ) === 0 ) {
// Safe to use
}
current_user_can()
Checks user permissions to ensure the user is authorized to perform an action.
if ( current_user_can( 'edit_posts' ) ) {
// Allow access
}
Implementing Sanitization and Validation in WordPress Plugins
Processing Form Submissions
When handling form submissions, always sanitize and validate inputs before processing.
Example:
if ( isset( $_POST['submit_form'] ) ) {
// Sanitize inputs
$user_name = sanitize_text_field( $_POST['user_name'] );
$user_email = sanitize_email( $_POST['user_email'] );
// Validate inputs
if ( ! is_email( $user_email ) ) {
wp_die( 'Invalid email address.' );
}
// Proceed with sanitized and validated data
// Save to database or perform other actions
}
Adding Settings to the Admin Dashboard
When creating settings pages, ensure all inputs are sanitized and validated before saving them to the database.
Example:
add_action( 'admin_init', 'myplugin_register_settings' );
function myplugin_register_settings() {
register_setting( 'myplugin_options_group', 'myplugin_option_name', 'myplugin_sanitize_option' );
}
function myplugin_sanitize_option( $input ) {
return sanitize_text_field( $input );
}
Securing Database Interactions
Using Prepared Statements
Use $wpdb
with prepared statements to prevent SQL injection.
global $wpdb;
$wpdb->insert(
$wpdb->prefix . 'custom_table',
array(
'user_name' => sanitize_text_field( $_POST['user_name'] ),
'user_email' => sanitize_email( $_POST['user_email'] ),
)
);
Escaping Outputs
Always escape data before outputting it to the browser to prevent XSS attacks.
Example:
echo esc_html( $user_name );
echo esc_url( $website_url );
Testing and Debugging Input Handling
Debugging Tools
Use tools like Query Monitor to inspect inputs and database interactions.
Install Query Monitor: Query Monitor Plugin
Error Logging
Enable error logging in wp-config.php
:
define( 'WP_DEBUG', true );
define( 'WP_DEBUG_LOG', true );
Review the logs in wp-content/debug.log
.
Best Practices for Sanitization and Validation
- Never Trust User Input: Treat all inputs as untrusted until sanitized and validated.
- Use WordPress Functions: Always use built-in WordPress functions for sanitization and validation.
- Escape Before Output: Sanitize and validate when saving data; escape when displaying it.
- Check User Permissions: Validate user capabilities with
current_user_can()
.
Tools for Testing and Validation
- PHP_CodeSniffer: Enforce WordPress coding standards, including input sanitization.
Visit PHP_CodeSniffer. - WP-CLI: Test database interactions and validate plugin behavior.
Visit WP-CLI Documentation.
Conclusion
Understanding how to sanitize validate user inputs is a fundamental skill for WordPress plugin developers. By implementing proper sanitization and validation techniques, you ensure your plugins are secure, functional, and compatible with WordPress best practices.
Incorporate these methods into your development workflow to safeguard your plugins from vulnerabilities and enhance user trust. For more detailed guidance, visit the WordPress Plugin Developer Handbook. With these tools and techniques, you can build reliable, secure plugins that stand the test of time.