Custom APIs are powerful tools that allow WordPress plugins to communicate with external services or provide unique functionality within a website. By developing custom APIs for plugins, you can enhance interactivity, integrate third-party tools, or enable data sharing between applications.
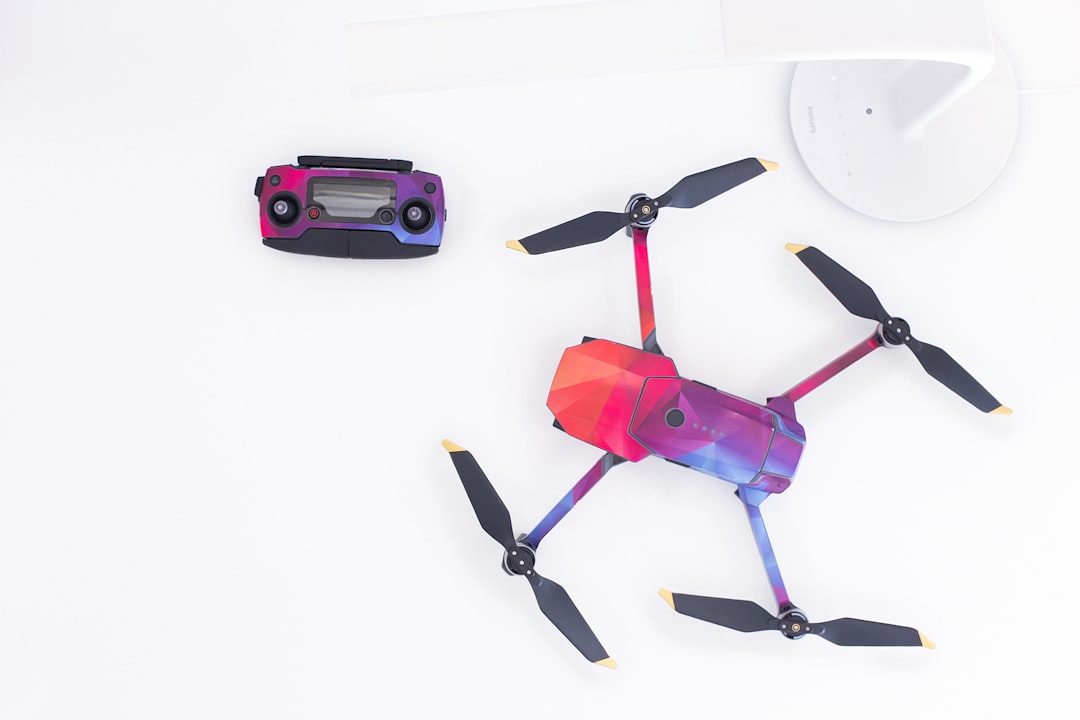
This guide will walk you through the basics of creating and implementing APIs in plugins, best practices for secure API development, and tips to optimize performance.
Why Use Custom APIs for Plugins
Custom APIs enable your plugin to provide dynamic, scalable features that enhance user experience. Here’s why they’re essential:
- Integration with External Services: APIs allow plugins to interact with payment gateways, CRMs, social media platforms, and more.
- Dynamic Content Updates: Custom APIs can fetch and display real-time data without requiring full-page reloads.
- Mobile App Integration: APIs enable mobile apps to access and interact with your WordPress site seamlessly.
- Data Sharing Across Applications: APIs provide a standard way to exchange data with other applications or platforms.
Focusing on custom APIs for plugins ensures your plugin is versatile, scalable, and ready for modern web development needs.
Setting Up Custom APIs for Plugins
Creating a custom API for your plugin involves registering endpoints, handling requests, and returning appropriate responses.
Register API Endpoints
Use the register_rest_route()
function to create custom REST API endpoints.
Example: Registering a custom endpoint:
add_action('rest_api_init', function() {
register_rest_route('myplugin/v1', '/data', array(
'methods' => 'GET',
'callback' => 'myplugin_get_data',
));
});
function myplugin_get_data() {
$data = array('message' => 'Hello, API!');
return rest_ensure_response($data);
}
In this example, the endpoint /wp-json/myplugin/v1/data
returns a JSON response containing a simple message.
Handle Parameters
Custom APIs often require query or body parameters. Use the $request
parameter to handle these inputs.
Example: Handling query parameters:
function myplugin_get_data_with_params($request) {
$param = $request->get_param('example');
return rest_ensure_response(array('received' => $param));
}
register_rest_route('myplugin/v1', '/data-with-params', array(
'methods' => 'GET',
'callback' => 'myplugin_get_data_with_params',
));
In this case, the API can accept a query parameter like example=value
.
Return Responses
Always return data in a JSON format using the rest_ensure_response()
function. This ensures proper formatting and compatibility with REST standards.
For more details, visit the WordPress REST API Handbook.
Custom APIs for Plugins and External Integrations
Custom APIs are invaluable for integrating third-party services and extending your plugin’s functionality.
Fetching External Data
Use WordPress’s HTTP API functions (wp_remote_get
or wp_remote_post
) to retrieve data from external APIs.
Example: Fetching data from an external API:
function myplugin_fetch_external_data() {
$response = wp_remote_get('https://api.example.com/data');
if (is_wp_error($response)) {
return rest_ensure_response(array('error' => 'Unable to fetch data.'));
}
$body = wp_remote_retrieve_body($response);
return rest_ensure_response(json_decode($body, true));
}
register_rest_route('myplugin/v1', '/external-data', array(
'methods' => 'GET',
'callback' => 'myplugin_fetch_external_data',
));
Sending Data to External Services
For POST requests, use wp_remote_post()
to send data to external services.
Example: Sending form data:
function myplugin_send_data($request) {
$data = array('field1' => $request->get_param('field1'));
$response = wp_remote_post('https://api.example.com/submit', array(
'body' => $data
));
if (is_wp_error($response)) {
return rest_ensure_response(array('error' => 'Submission failed.'));
}
return rest_ensure_response(array('success' => 'Data submitted successfully.'));
}
register_rest_route('myplugin/v1', '/submit-data', array(
'methods' => 'POST',
'callback' => 'myplugin_send_data',
));
For additional guidance, explore WPBeginner’s REST API Tutorial.
Securing Custom APIs for Plugins
Security is a critical aspect of developing custom APIs for plugins, as they expose data and functionality to external systems.
Use Nonces for Authentication
Nonces provide a secure way to verify requests. Use wp_create_nonce()
and check_ajax_referer()
for client-side and server-side validation.
Example: Verifying a nonce:
function myplugin_secure_endpoint($request) {
$nonce = $request->get_header('X-WP-Nonce');
if (!wp_verify_nonce($nonce, 'myplugin_nonce')) {
return new WP_Error('unauthorized', 'Unauthorized request', array('status' => 401));
}
return rest_ensure_response(array('success' => 'Authorized request'));
}
Restrict Endpoint Access
Restrict certain endpoints to authenticated users by setting the permission_callback
parameter.
Example: Restricting to logged-in users:
register_rest_route('myplugin/v1', '/restricted', array(
'methods' => 'GET',
'callback' => 'myplugin_restricted_data',
'permission_callback' => function() {
return is_user_logged_in();
}
));
Validate and Sanitize Inputs
Always sanitize user inputs and validate them before processing.
Example:
$sanitized = sanitize_text_field($request->get_param('example'));
For more security tips, visit Sucuri’s WordPress Security Blog.
Optimizing Custom APIs for Plugins
Efficient APIs ensure better performance and scalability.
Cache API Responses
Avoid unnecessary processing by caching responses using the Transients API.
Example:
function myplugin_cached_data() {
$cached_data = get_transient('myplugin_api_cache');
if (!$cached_data) {
$cached_data = array('message' => 'Hello, API!');
set_transient('myplugin_api_cache', $cached_data, HOUR_IN_SECONDS);
}
return rest_ensure_response($cached_data);
}
Minimize Query Load
Optimize database queries in API callbacks to prevent server overload. Use indexed queries and LIMIT
where possible.
Use Pagination for Large Datasets
For endpoints returning large datasets, implement pagination to reduce load times.
Example:
$args = array(
'post_type' => 'post',
'posts_per_page' => $request->get_param('per_page') ?? 10,
'paged' => $request->get_param('page') ?? 1
);
$query = new WP_Query($args);
return rest_ensure_response($query->posts);
For advanced optimization tips, visit Kinsta’s API Optimization Guide.
Testing and Debugging Custom APIs
Testing ensures your API endpoints function as intended and handle edge cases gracefully.
Use API Testing Tools
Tools like Postman or Insomnia make it easy to test endpoints.
Log Requests and Responses
Log API requests and responses for debugging purposes using error_log()
.
Simulate Real-World Scenarios
Test your API under conditions like high traffic, varied parameters, and unexpected inputs.
Conclusion
Custom APIs for plugins unlock endless possibilities for extending WordPress functionality, enabling dynamic content, third-party integrations, and seamless user experiences. By adhering to best practices for security, optimization, and testing, you can create reliable and efficient APIs.
Start developing custom APIs for plugins today to deliver robust and scalable solutions for your clients or users. For more resources, explore the WordPress REST API Handbook or Smashing Magazine’s API Design Guide.