Custom post types are one of the most powerful features of WordPress, allowing developers to create specialized content types beyond the default posts and pages. When packaged into plugins, custom post types become a flexible and reusable solution for adding unique content structures to WordPress websites. This guide covers everything you need to know about developing custom post types plugins, from understanding the basics to implementing best practices.
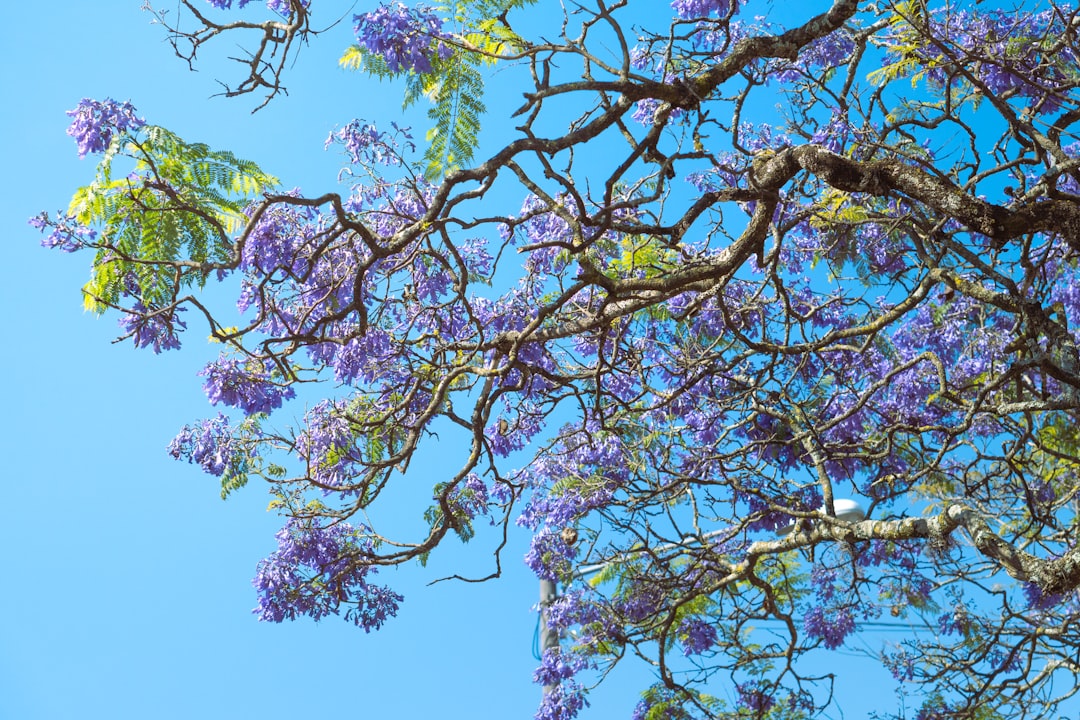
Why Use Custom Post Types Plugins
Custom post types (CPTs) extend WordPress’s functionality by enabling the creation of tailored content formats. With custom post types plugins, developers can:
- Organize Content Efficiently: Create separate sections for portfolios, testimonials, events, products, or any custom content.
- Improve User Experience: Provide a structured backend for site administrators to manage custom content effortlessly.
- Enhance Reusability: Package CPT functionality into plugins for deployment across multiple projects.
- Ensure Theme Independence: Keep CPTs in plugins to prevent content loss when switching themes.
By focusing on custom post-type plugins, you provide users with scalable and maintainable solutions for managing diverse content types.
Setting Up CPTs in Plugins
Developing a plugin with custom post types requires setting up the plugin structure, registering CPTs, and customizing their behavior.
Plugin Initialization
Start by creating a new directory in the wp-content/plugins
folder and adding a main plugin file.
Example: custom-post-type-plugin/custom-post-type-plugin.php
:
<?php
/**
* Plugin Name: Custom Post Types Plugin
* Description: A plugin to add custom post types to WordPress.
* Version: 1.0
* Author: Your Name
*/
if (!defined('ABSPATH')) {
exit; // Exit if accessed directly
}
Register Custom Post Types
Use the register_post_type()
function to define a new custom post type.
Example: Adding a “Portfolio” post type:
function create_portfolio_post_type() {
$labels = array(
'name' => __('Portfolios'),
'singular_name' => __('Portfolio'),
'add_new' => __('Add New Portfolio'),
'edit_item' => __('Edit Portfolio'),
'all_items' => __('All Portfolios')
);
$args = array(
'labels' => $labels,
'public' => true,
'has_archive' => true,
'supports' => array('title', 'editor', 'thumbnail', 'custom-fields'),
'menu_icon' => 'dashicons-portfolio'
);
register_post_type('portfolio', $args);
}
add_action('init', 'create_portfolio_post_type');
Add Custom Taxonomies
Enhance your CPT by associating custom taxonomies using register_taxonomy()
.
Example: Adding a “Project Type” taxonomy to the “Portfolio” post type:
function create_project_type_taxonomy() {
$labels = array(
'name' => __('Project Types'),
'singular_name' => __('Project Type'),
'search_items' => __('Search Project Types'),
'all_items' => __('All Project Types')
);
$args = array(
'hierarchical' => true,
'labels' => $labels,
'show_ui' => true,
'show_in_menu' => true
);
register_taxonomy('project_type', 'portfolio', $args);
}
add_action('init', 'create_project_type_taxonomy');
Custom Post Types Plugins for Specialized Content
Custom post types of plugins can be used to create tailored solutions for various use cases.
Building a Portfolio Plugin
A portfolio plugin can showcase creative work, complete with categories, tags, and custom fields for project details.
Enhance the “Portfolio” post type by adding meta boxes:
function add_portfolio_meta_boxes() {
add_meta_box(
'portfolio_details',
__('Portfolio Details'),
'portfolio_meta_box_callback',
'portfolio'
);
}
add_action('add_meta_boxes', 'add_portfolio_meta_boxes');
function portfolio_meta_box_callback($post) {
$client_name = get_post_meta($post->ID, '_client_name', true);
echo '<label for="client_name">' . __('Client Name:') . '</label>';
echo '<input type="text" id="client_name" name="client_name" value="' . esc_attr($client_name) . '" />';
}
function save_portfolio_meta($post_id) {
if (array_key_exists('client_name', $_POST)) {
update_post_meta($post_id, '_client_name', sanitize_text_field($_POST['client_name']));
}
}
add_action('save_post', 'save_portfolio_meta');
Creating an Events Plugin
An events plugin might include fields for date, time, location, and registration links. Use custom fields or advanced custom fields (ACF) for these features.
For a complete solution, explore The Events Calendar plugin.
Optimizing CPT Plugins for Performance
Well-optimized plugins ensure your CPT functionality doesn’t slow down the website.
Optimize Queries
Use the WP_Query
class for efficient database queries.
Example: Fetching recent portfolio posts:
$args = array(
'post_type' => 'portfolio',
'posts_per_page' => 5
);
$query = new WP_Query($args);
if ($query->have_posts()) {
while ($query->have_posts()) {
$query->the_post();
the_title();
}
wp_reset_postdata();
}
Lazy Load Assets
Load JavaScript and CSS conditionally to reduce unnecessary resource usage.
Example:
function load_portfolio_assets($hook) {
if ('edit.php?post_type=portfolio' === $hook) {
wp_enqueue_style('portfolio-styles', plugin_dir_url(__FILE__) . 'css/portfolio.css');
}
}
add_action('admin_enqueue_scripts', 'load_portfolio_assets');
Cache Content
Use caching plugins or implement transient API to reduce repeated database queries.
Learn more about optimization at Kinsta’s Performance Guide.
Best Practices for CPT Plugins
Follow these best practices to create efficient and maintainable plugins:
Separate Plugin and Theme Functionality
Keep CPTs in plugins instead of themes to prevent data loss when switching themes.
Use Descriptive Naming Conventions
Name your CPTs and functions uniquely to avoid conflicts with other plugins or themes.
Provide Documentation
Document your plugin’s features and usage instructions for developers and users.
Test for Compatibility
Ensure your plugin works well with other plugins and popular themes to avoid conflicts.
Testing and Debugging Custom Post Types Plugins
Testing ensures your plugin performs reliably under different conditions.
Test in Staging Environments
Use staging sites to test CPT functionality without affecting live websites.
Debug Errors
Enable WordPress debugging to identify issues during development:
define('WP_DEBUG', true);
define('WP_DEBUG_LOG', true);
Validate Data
Use WordPress functions like sanitize_text_field
and esc_html
to validate and escape user inputs.
Conclusion
Custom post types plugins provide the foundation for creating tailored content structures that meet unique user needs. By following best practices and focusing on performance optimization, you can develop plugins that are both functional and scalable.
Start building custom post-type plugins today and deliver powerful content management solutions to your clients or users. For further resources, explore the WordPress Plugin Developer Handbook or Smashing Magazine’s Guide to WordPress Custom Post Types.