REST APIs have revolutionized how applications interact, enabling seamless data sharing and integration across platforms. In the WordPress ecosystem, REST APIs allow developers to build robust plugins that communicate with external services or provide dynamic content to applications. Mastering REST API plugin development is crucial for creating scalable and versatile solutions.
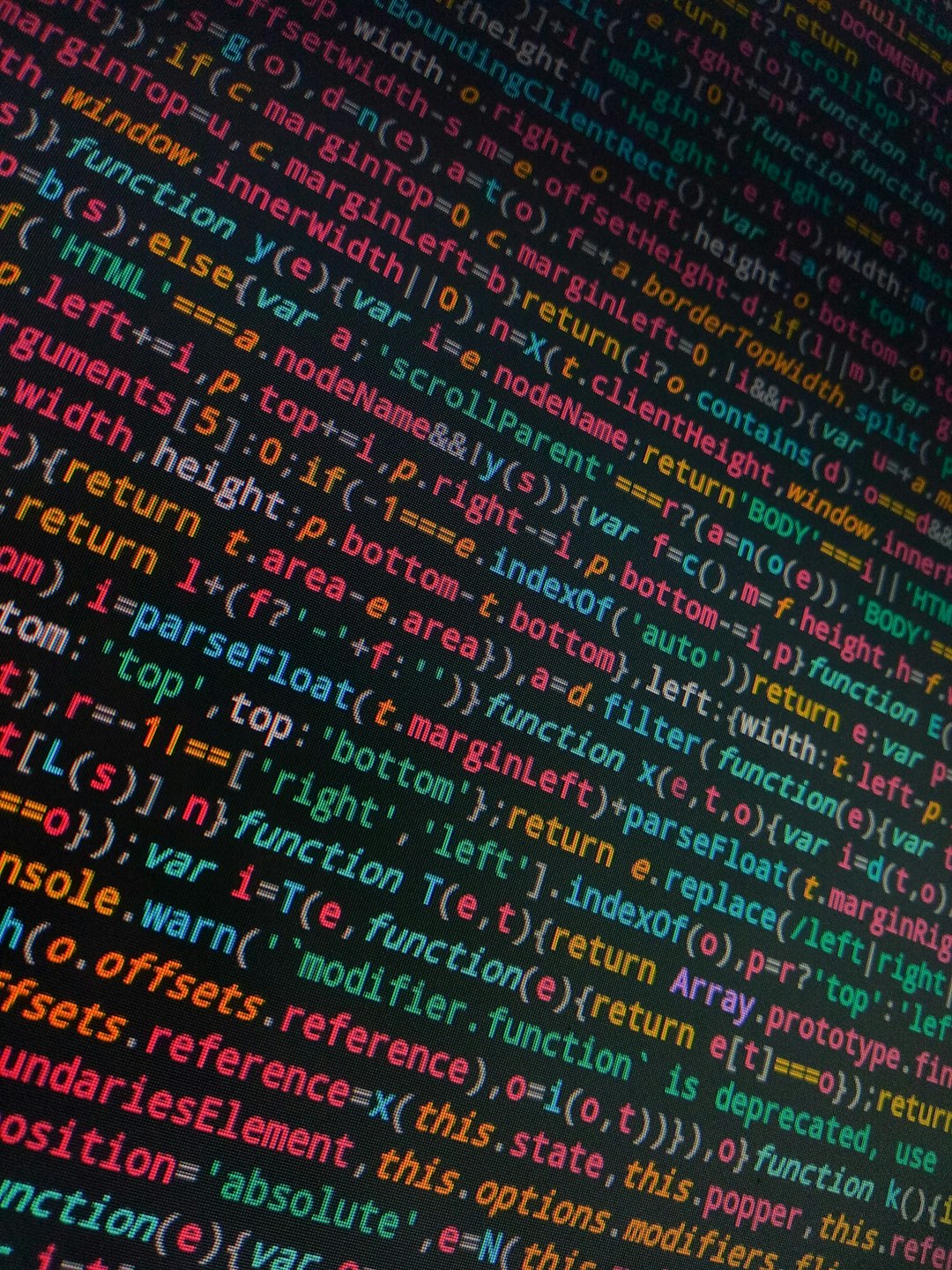
This guide explores the essential steps, best practices, and tools for developing plugins with REST API functionality.
Why REST API Plugins Development is Important
REST API plugins empower developers to extend WordPress functionality beyond traditional boundaries. Here’s why REST API plugins development matters:
- Enhanced Integration: APIs enable plugins to connect with third-party services like CRMs, payment gateways, or social media platforms.
- Dynamic Applications: REST APIs allow real-time updates without full-page reloads, improving user experience.
- Cross-Platform Access: APIs make it easy to integrate WordPress with mobile apps, SPAs (Single Page Applications), and external systems.
- Scalable Architecture: REST APIs decouple data from presentation, making plugins flexible and reusable.
By leveraging REST APIs, you can create powerful plugins that enhance WordPress functionality and user experience.
Setting Up REST API Endpoints in Plugins
Developing a plugin with REST API functionality involves registering custom endpoints, handling requests, and returning JSON responses.
Registering REST API Endpoints
Use the register_rest_route()
function to define custom endpoints.
Example: Adding a custom endpoint:
add_action('rest_api_init', function() {
register_rest_route('myplugin/v1', '/items', array(
'methods' => 'GET',
'callback' => 'myplugin_get_items',
));
});
function myplugin_get_items() {
$data = array('item1', 'item2', 'item3');
return rest_ensure_response($data);
}
This example creates an endpoint accessible at /wp-json/myplugin/v1/items
, which returns a JSON array of items.
Handling Parameters
APIs often require query or body parameters to filter or manipulate data. Use the $request
parameter to access inputs.
Example: Handling query parameters:
function myplugin_get_filtered_items($request) {
$category = $request->get_param('category');
$items = array_filter(get_items(), function($item) use ($category) {
return $item['category'] === $category;
});
return rest_ensure_response($items);
}
register_rest_route('myplugin/v1', '/filtered-items', array(
'methods' => 'GET',
'callback' => 'myplugin_get_filtered_items',
));
Returning JSON Responses
Ensure all responses are returned in JSON format using the rest_ensure_response()
function. This ensures compatibility with REST standards and simplifies client-side processing.
For a detailed overview, visit the WordPress REST API Handbook.
REST API Plugins Development for Third-Party Integrations
REST APIs are invaluable for integrating WordPress plugins with external services.
Fetching Data from External APIs
Use WordPress’s HTTP API functions, such as wp_remote_get
, to fetch data from external sources.
Example: Fetching data from an external API:
function myplugin_fetch_external_data() {
$response = wp_remote_get('https://api.example.com/data');
if (is_wp_error($response)) {
return rest_ensure_response(array('error' => 'Unable to fetch data'));
}
$data = json_decode(wp_remote_retrieve_body($response), true);
return rest_ensure_response($data);
}
register_rest_route('myplugin/v1', '/external-data', array(
'methods' => 'GET',
'callback' => 'myplugin_fetch_external_data',
));
Sending Data to External APIs
Use wp_remote_post
to send data to external APIs, enabling two-way communication.
Example: Sending form data:
function myplugin_send_data($request) {
$data = array('name' => $request->get_param('name'));
$response = wp_remote_post('https://api.example.com/submit', array(
'body' => $data
));
if (is_wp_error($response)) {
return rest_ensure_response(array('error' => 'Submission failed'));
}
return rest_ensure_response(array('success' => 'Data submitted successfully'));
}
register_rest_route('myplugin/v1', '/submit-data', array(
'methods' => 'POST',
'callback' => 'myplugin_send_data',
));
For additional insights, explore WPBeginner’s REST API Integration Guide.
Securing REST API Plugins
Security is a top priority in REST API plugin development, as APIs expose functionality to external systems.
Authenticate API Requests
Use nonces or token-based authentication to secure API endpoints.
Example: Verifying a nonce:
function myplugin_secure_endpoint($request) {
$nonce = $request->get_header('X-WP-Nonce');
if (!wp_verify_nonce($nonce, 'myplugin_nonce')) {
return new WP_Error('unauthorized', 'Unauthorized request', array('status' => 401));
}
return rest_ensure_response(array('success' => 'Authorized request'));
}
Restrict Access
Restrict sensitive endpoints to authenticated users using the permission_callback
parameter.
Example: Restricting to logged-in users:
register_rest_route('myplugin/v1', '/protected-data', array(
'methods' => 'GET',
'callback' => 'myplugin_get_protected_data',
'permission_callback' => function() {
return is_user_logged_in();
}
));
Sanitize and Validate Inputs
Sanitize and validate user inputs to prevent injection attacks.
Example:
$sanitized_param = sanitize_text_field($request->get_param('example'));
For more security best practices, visit Sucuri’s Security Blog.
Optimizing REST API Plugins
Efficient APIs improve performance and scalability, ensuring a smooth user experience.
Cache API Responses
Reduce server load by caching API responses using the Transients API.
Example:
function myplugin_cached_endpoint() {
$cached_data = get_transient('myplugin_cache');
if (!$cached_data) {
$cached_data = array('message' => 'Fresh API Response');
set_transient('myplugin_cache', $cached_data, HOUR_IN_SECONDS);
}
return rest_ensure_response($cached_data);
}
Paginate Large Datasets
Implement pagination to return large datasets efficiently.
Example:
$args = array(
'post_type' => 'post',
'posts_per_page' => $request->get_param('per_page') ?? 10,
'paged' => $request->get_param('page') ?? 1
);
$query = new WP_Query($args);
return rest_ensure_response($query->posts);
For performance optimization tips, visit Kinsta’s REST API Guide.
Testing and Debugging REST API Plugins
Thorough testing ensures your REST API endpoints are reliable and secure.
Use API Testing Tools
Tools like Postman or Insomnia simplify endpoint testing.
Enable Debugging
Log requests and responses for debugging purposes using error_log()
.
Simulate Real-World Scenarios
Test your API under conditions like high traffic, varied inputs, and authentication errors to ensure robustness.
Conclusion
REST API plugins open a world of possibilities for WordPress development, enabling dynamic interactions, seamless integrations, and scalable architectures. By mastering REST API plugin development, you can build powerful tools that enhance website functionality and user experience.
Start creating your API-powered plugins today and explore additional resources like the WordPress REST API Handbook or Smashing Magazine’s REST API Guide.