WordPress hooks are an essential feature of the platform that allows developers to modify or extend its functionality without altering the core files. Understanding and effectively utilizing WordPress hooks in plugin development is key to creating flexible, maintainable, and feature-rich plugins.
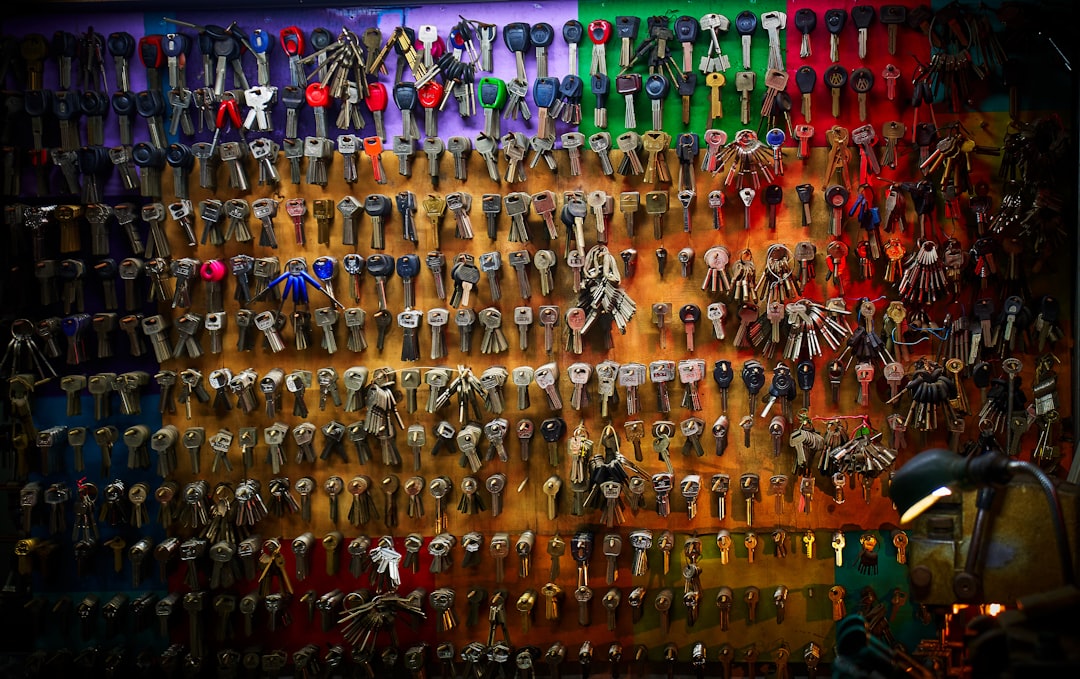
This guide explores the two main types of WordPress hooks—actions and filters—how they work, and best practices for integrating them into your plugin development workflow.
What Are WordPress Hooks?
WordPress hooks are predefined points in the WordPress core that allow developers to insert custom code or modify existing behavior. They provide a safe and efficient way to extend WordPress functionality.
- Actions: Allow you to execute custom code at specific points, such as when a post is published or a user logs in.
- Filters: Enables you to modify data before it is displayed or processed, such as changing the content of a post or customizing a query.
By leveraging WordPress hooks in plugin development, you can create plugins that seamlessly integrate with WordPress and other plugins.
Learn more about hooks in the WordPress Plugin Handbook.
Using Actions in Plugin Development
Actions are one of the two primary types of hooks in WordPress. They allow you to add custom functionality at predefined points during the WordPress lifecycle.
Adding Custom Actions
To use an action, you attach a custom function to a specific action hook using add_action
.
Example:
add_action('wp_head', 'add_custom_meta_tag');
function add_custom_meta_tag() {
echo '<meta name="custom-meta" content="Plugin Example">';
}
This code adds a custom meta tag to the <head>
section of your site.
Common Action Hooks
Some commonly used action hooks include:
init
: Triggered after WordPress has loaded but before the output is sent.wp_enqueue_scripts
: Used to enqueue scripts and styles.save_post
: Fires when a post is saved, allowing custom processing of post data.
Explore a complete list of action hooks on the WordPress Developer Reference.
Using Filters in Plugin Development
Filters allow you to modify data as it is being processed or displayed, providing granular control over WordPress output.
Adding Custom Filters
To use a filter, you attach a custom function to a filter hook using add_filter
.
Example:
add_filter('the_content', 'custom_content_filter');
function custom_content_filter($content) {
$custom_text = '<p>This is added by the plugin.</p>';
return $custom_text . $content;
}
This code prepends a custom message to all post content.
Common Filter Hooks
Popular filter hooks include:
the_content
: Modify the content of posts and pages.wp_title
: Customize the page title.login_message
: Add a custom message to the login screen.
For more examples, visit the WordPress Filter Reference.
WordPress Hooks in Plugin Development for Custom Functionality
Using hooks effectively enables you to add unique features and extend WordPress functionality in creative ways.
Create Custom Post Types with Hooks
Hooks like init
can be used to register custom post types programmatically.
Example:
add_action('init', 'register_custom_post_type');
function register_custom_post_type() {
register_post_type('custom_type', array(
'label' => 'Custom Type',
'public' => true,
'supports' => array('title', 'editor', 'thumbnail'),
));
}
This code creates a custom post type called “Custom Type.”
Add Custom User Roles
Modify user roles and capabilities using the init
action.
Example:
add_action('init', 'add_custom_user_role');
function add_custom_user_role() {
add_role('custom_role', 'Custom Role', array(
'read' => true,
'edit_posts' => false,
));
}
This code adds a new user role with specific permissions.
Explore more examples of custom post types and roles in the WordPress Plugin Handbook.
Best Practices for Using WordPress Hooks
While hooks are powerful, improper usage can lead to conflicts, performance issues, or unexpected behavior. Follow these best practices for optimal results.
Prefix Hook Names
When creating custom hooks in your plugin, prefix the hook names with your plugin’s name to avoid conflicts with other plugins.
Example:
do_action('myplugin_custom_action');
Prioritize and Modify Hook Order
Use the priority parameter in add_action
or add_filter
to control the order in which hooks execute.
Example:
add_action('init', 'custom_init_action', 20);
The default priority is 10, and higher numbers run later.
Always Return Data in Filters
Filters must return the modified data; otherwise, they may disrupt functionality.
Example:
add_filter('the_title', 'custom_title_filter');
function custom_title_filter($title) {
return 'Custom: ' . $title;
}
Use Hook Removal with Care
If necessary, you can remove a hook using remove_action
or remove_filter
. However, avoid excessive use as it may lead to conflicts.
Example:
remove_action('wp_head', 'wp_generator');
Learn more about best practices in Smashing Magazine’s WordPress Hooks Guide.
Debugging WordPress Hooks
Debugging hooks is essential to ensure your plugin behaves as expected.
Use the doing_action
or doing_filter
Functions
These functions help determine if a specific hook is currently running.
Example:
if (doing_action('wp_head')) {
error_log('The wp_head action is running.');
}
Use Debugging Plugins
Plugins like Query Monitor allow you to track hook execution, monitor performance, and debug issues in your plugin.
Log Hook Output
Use error_log
to log information about hook behavior for debugging purposes.
Example:
add_action('init', function() {
error_log('Init hook fired.');
});
Conclusion
WordPress hooks are the foundation of plugin development, enabling developers to create dynamic and feature-rich plugins while maintaining compatibility with the WordPress core. By mastering actions and filters, and following best practices, you can build plugins that integrate seamlessly and perform efficiently.
Start applying WordPress hooks in plugin development to your projects today, and explore additional resources like the WordPress Developer Handbook or Codeable’s Plugin Development Services.