In the world of modern web development, interactivity and responsiveness are critical for providing a seamless user experience. AJAX (Asynchronous JavaScript and XML) is a powerful tool for creating dynamic behavior without requiring full-page reloads. For WordPress developers, understanding how to implement AJAX functionality in plugins can take plugin development to the next level, enabling features like real-time search, form submission, or live data updates.
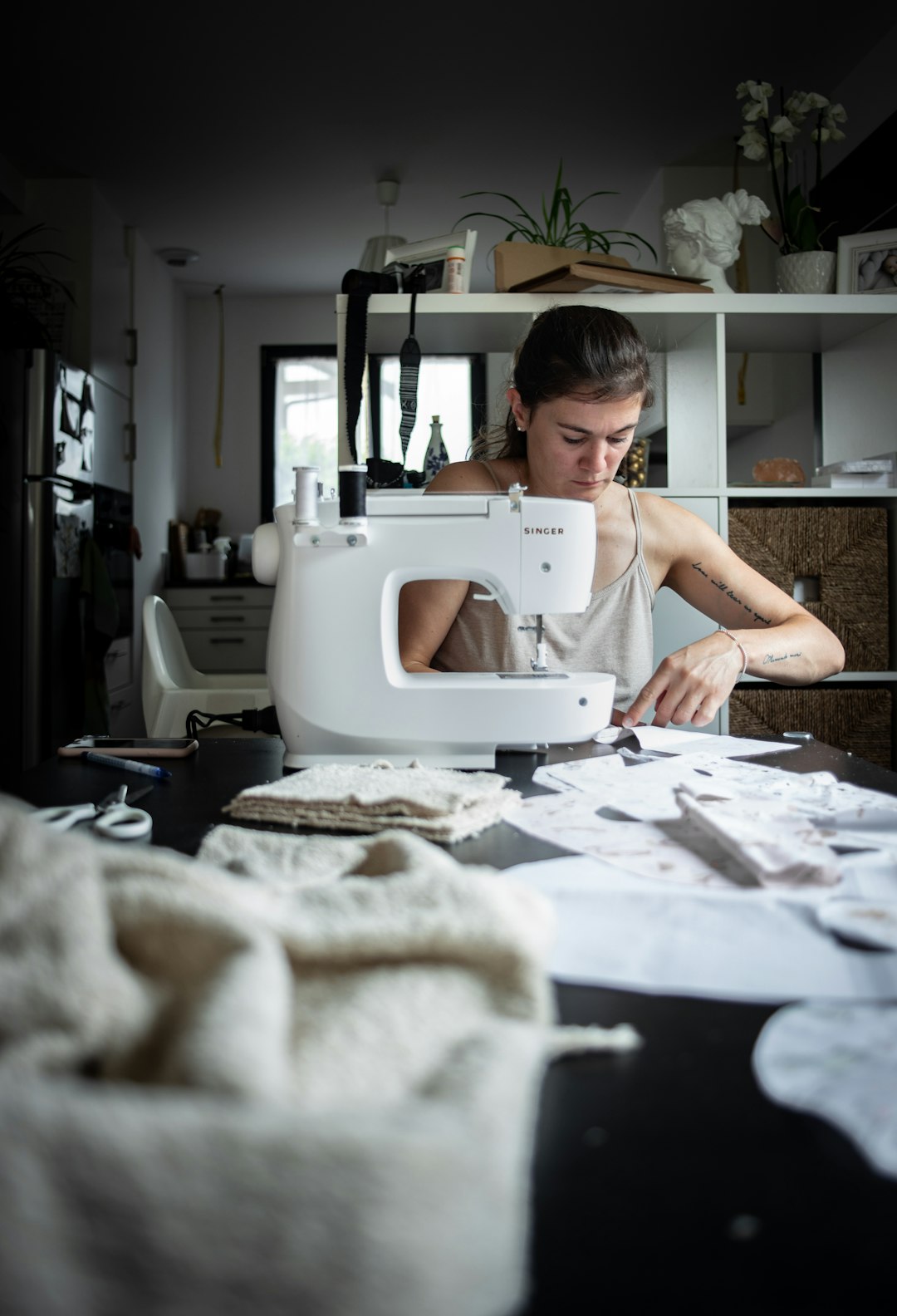
This guide explores the fundamentals of AJAX, its integration into WordPress plugins, and best practices for implementing dynamic behavior efficiently.
What Is AJAX and Why Use It?
AJAX allows web applications to communicate with the server asynchronously, meaning users can interact with your website without waiting for a full-page reload. This enhances the overall user experience by making interactions faster and smoother.
Benefits of AJAX in plugins include:
- Improved Interactivity: Enables real-time updates, such as live comments or instant search results.
- Faster Load Times: Reduces server load by only fetching specific data instead of reloading entire pages.
- Enhanced User Experience: Provides seamless interactions, keeping users engaged.
For more on AJAX, check out Mozilla’s AJAX Guide.
How WordPress Handles AJAX
In WordPress, AJAX requests are processed through the admin-ajax.php
file. This file acts as a central endpoint for handling AJAX calls. Here’s how it works:
- A JavaScript function sends a request to
admin-ajax.php
. - WordPress processes the request using a PHP function linked to an AJAX action hook.
- The PHP function generates a response, which is sent back to the JavaScript function.
- The JavaScript function handles the response and updates the page dynamically.
Setting Up AJAX Functionality in Plugins
To implement AJAX functionality in plugins, follow these steps:
Enqueue Scripts
The first step is to enqueue the JavaScript file responsible for sending the AJAX request.
Example:
add_action('wp_enqueue_scripts', 'enqueue_ajax_script');
function enqueue_ajax_script() {
wp_enqueue_script('my-plugin-ajax', plugin_dir_url(__FILE__) . 'js/ajax.js', array('jquery'), null, true);
wp_localize_script('my-plugin-ajax', 'ajax_object', array(
'ajax_url' => admin_url('admin-ajax.php'),
'nonce' => wp_create_nonce('my_plugin_nonce')
));
}
Here, wp_localize_script
passes the AJAX URL and a nonce for security to the JavaScript file.
Create the JavaScript File
In your JavaScript file, write the function to send the AJAX request.
Example:
jQuery(document).ready(function($) {
$('#my-button').on('click', function() {
$.ajax({
url: ajax_object.ajax_url,
type: 'POST',
data: {
action: 'my_custom_action',
nonce: ajax_object.nonce,
value: $('#my-input').val()
},
success: function(response) {
$('#response-container').html(response);
},
error: function(error) {
console.log('Error:', error);
}
});
});
});
Add the PHP Callback
Create a PHP function to handle the AJAX request and link it to an action hook.
Example:
add_action('wp_ajax_my_custom_action', 'handle_ajax_request');
add_action('wp_ajax_nopriv_my_custom_action', 'handle_ajax_request');
function handle_ajax_request() {
check_ajax_referer('my_plugin_nonce', 'nonce');
$value = sanitize_text_field($_POST['value']);
echo 'You entered: ' . $value;
wp_die(); // Ends the AJAX request.
}
Here, wp_ajax_my_custom_action
is for logged-in users, and wp_ajax_nopriv_my_custom_action
handles requests from non-logged-in users.
Learn more about AJAX in WordPress on the WordPress Developer Handbook.
AJAX Functionality in Plugins for Real-Time Search
Real-time search is one of the most popular use cases for AJAX. It allows users to see search results instantly as they type, improving usability and engagement.
Create the Search Form
Add a search form to your plugin’s front end.
Example:
<form id="search-form">
<input type="text" id="search-input" placeholder="Search...">
<div id="search-results"></div>
</form>
Handle the Search Request
In your JavaScript file, send the search query to the server using AJAX.
Example:
$('#search-input').on('keyup', function() {
$.ajax({
url: ajax_object.ajax_url,
type: 'POST',
data: {
action: 'search_posts',
nonce: ajax_object.nonce,
query: $(this).val()
},
success: function(response) {
$('#search-results').html(response);
}
});
});
Process the Search Query
Write a PHP function to query posts based on the search term.
Example:
add_action('wp_ajax_search_posts', 'handle_search_query');
add_action('wp_ajax_nopriv_search_posts', 'handle_search_query');
function handle_search_query() {
check_ajax_referer('my_plugin_nonce', 'nonce');
$query = sanitize_text_field($_POST['query']);
$args = array(
's' => $query,
'posts_per_page' => 5
);
$posts = get_posts($args);
if (!empty($posts)) {
foreach ($posts as $post) {
echo '<div>' . esc_html($post->post_title) . '</div>';
}
} else {
echo 'No results found.';
}
wp_die();
}
For advanced search functionality, consider integrating plugins like Relevanssi.
Best Practices for AJAX Functionality in Plugins
While AJAX adds significant value, improper implementation can lead to performance issues or security vulnerabilities. Follow these best practices:
Validate and Sanitize Inputs
Always validate and sanitize user input to prevent SQL injection and other attacks. Use functions like sanitize_text_field
and esc_sql
.
Use Nonces for Security
Protect AJAX requests with nonces to verify their authenticity. Use wp_create_nonce
to generate nonces and check_ajax_referer
to validate them.
Optimize Database Queries
Avoid excessive or unoptimized queries in your AJAX callbacks. Use caching when possible to reduce server load.
Minimize JavaScript Bloat
Keep your JavaScript file concise and modular to ensure fast loading times. Consider bundling and minifying scripts using tools like Webpack.
Explore additional security measures for AJAX on Sucuri’s Blog.
Debugging AJAX in WordPress Plugins
Debugging AJAX can be challenging due to its asynchronous nature. Use the following techniques to troubleshoot issues:
Log Errors
Use console.log
in JavaScript and error_log
in PHP to identify issues in your code.
Test with Tools
Use browser developer tools to monitor AJAX requests and responses. Tools like Postman can also help simulate AJAX requests.
Check Server Errors
Inspect your server’s error logs for issues that might not be immediately visible in the browser console.
Conclusion
Implementing AJAX functionality in plugins allows you to create dynamic and responsive features that enhance user experience and make your plugins stand out. From real-time search to interactive forms, AJAX provides endless possibilities for modern web development.
By following best practices, optimizing performance, and ensuring security, you can integrate AJAX into your WordPress plugins efficiently and effectively. For more resources, explore the WordPress Plugin Developer Handbook or MDN Web Docs on AJAX.