Developing WordPress plugins tailored to meet individual client needs is a valuable skill for web developers. Custom plugins allow you to deliver features that align precisely with a client’s business requirements, making their website more functional, efficient, and user-friendly. Client-specific plugin development involves creating bespoke solutions while ensuring scalability, maintainability, and security.
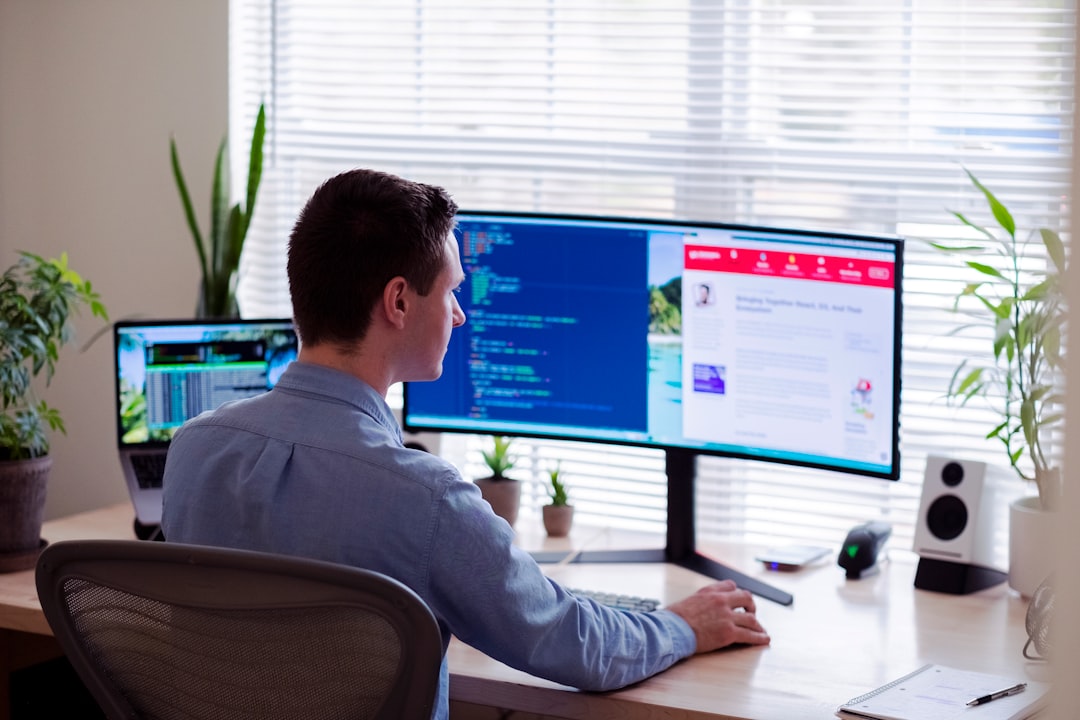
This guide explores the key steps, best practices, and tools needed to build plugins that cater to unique client demands.
Why Client-Specific Plugin Development Matters
While many plugins offer excellent functionality, they often lack the ability to address the unique needs of specific businesses. By focusing on client-specific plugin development, you provide:
- Tailored Solutions: Custom plugins solve business-specific challenges without unnecessary features.
- Improved Efficiency: Automation of processes tailored to the client’s workflow saves time and effort.
- Scalability: Custom plugins grow with the client’s needs, avoiding the limitations of off-the-shelf solutions.
- Competitive Advantage: Bespoke functionality can help clients stand out in their industry.
Investing in custom plugin development ensures your client receives a solution built specifically for their goals.
Setting Up a Development Environment
A solid development environment is the foundation of any successful plugin project.
Local Development
Develop your plugin locally to streamline the testing process and reduce deployment errors. Popular tools include:
- Local by Flywheel: A user-friendly local WordPress environment.
- XAMPP or MAMP: Cross-platform local servers for running WordPress.
- Docker: A containerized solution for managing development environments.
Version Control
Use Git to track changes and collaborate effectively. Platforms like GitHub or GitLab allow you to manage repositories and share code securely.
Debugging Tools
Enable debugging in your WordPress setup to catch errors early:
define('WP_DEBUG', true);
define('WP_DEBUG_LOG', true);
Plugins like Query Monitor can help identify performance bottlenecks and code issues.
For more setup tips, visit the WordPress Developer Handbook.
Key Steps in Client-Specific Plugin Development
Creating a plugin tailored to a client’s needs requires careful planning, execution, and testing.
Define the Requirements
Begin by gathering detailed requirements from your client. Conduct interviews or workshops to identify:
- Business goals.
- User pain points.
- Desired features and functionalities.
Document these needs in a project brief to ensure clarity and alignment.
Plan the Plugin Architecture
Design a scalable and maintainable architecture for your plugin. Include:
- Custom Post Types: For structured data storage.
- Shortcodes or Blocks: For frontend content display.
- Admin Settings Pages: For client configuration options.
Example: Adding a “Team Members” custom post type:
function create_team_members_post_type() {
$args = array(
'labels' => array(
'name' => __('Team Members'),
'singular_name' => __('Team Member')
),
'public' => true,
'supports' => array('title', 'editor', 'thumbnail'),
);
register_post_type('team_member', $args);
}
add_action('init', 'create_team_members_post_type');
Develop Core Features
Focus on core functionality before adding enhancements. Use WordPress hooks, filters, and APIs to integrate seamlessly with the platform.
For guidance on WordPress APIs, explore WPBeginner’s Developer Tutorials.
Client-Specific Plugin Development for Automation
Automation is a common requirement in client-specific plugin development. Custom plugins can streamline repetitive tasks and improve efficiency.
Automating Data Imports
Create a plugin that imports data from external sources, such as CRMs or APIs.
Example: Importing data via REST API:
function import_client_data() {
$response = wp_remote_get('https://api.example.com/data');
if (!is_wp_error($response)) {
$data = json_decode(wp_remote_retrieve_body($response), true);
foreach ($data as $item) {
wp_insert_post(array(
'post_type' => 'team_member',
'post_title' => sanitize_text_field($item['name']),
'post_content' => sanitize_text_field($item['bio']),
));
}
}
}
add_action('init', 'import_client_data');
Scheduling Cron Jobs
Automate recurring tasks like sending reports or updating data using WordPress Cron.
Example: Scheduling a weekly task:
function schedule_weekly_report() {
if (!wp_next_scheduled('generate_weekly_report')) {
wp_schedule_event(time(), 'weekly', 'generate_weekly_report');
}
}
add_action('wp', 'schedule_weekly_report');
function generate_weekly_report() {
// Generate and email the report
}
add_action('generate_weekly_report', 'generate_weekly_report');
For more on automation, visit Kinsta’s Cron Jobs Guide.
Optimizing Client-Specific Plugins for Performance
Performance optimization ensures your plugin runs smoothly without affecting the client’s site speed.
Minimize Database Queries
Avoid excessive or inefficient database queries. Use WP_Query
for optimal performance.
Example: Fetching posts with specific metadata:
$args = array(
'post_type' => 'team_member',
'meta_query' => array(
array(
'key' => 'role',
'value' => 'manager',
'compare' => '='
)
)
);
$query = new WP_Query($args);
Lazy Load Assets
Only load JavaScript and CSS files on pages where they’re needed.
Example:
function load_plugin_assets($hook) {
if ('settings_page_client_plugin' === $hook) {
wp_enqueue_style('plugin-styles', plugin_dir_url(__FILE__) . 'css/styles.css');
}
}
add_action('admin_enqueue_scripts', 'load_plugin_assets');
Cache Results
Use the Transients API to cache frequently accessed data.
Example:
function get_cached_data() {
$data = get_transient('cached_data');
if (!$data) {
$data = fetch_expensive_data();
set_transient('cached_data', $data, HOUR_IN_SECONDS);
}
return $data;
}
Learn more about optimization from Smashing Magazine’s WordPress Performance Guide.
Testing and Debugging Client-Specific Plugins
Thorough testing ensures the plugin works flawlessly across different environments.
Functional Testing
Test every feature against the client’s requirements. Use staging environments to simulate real-world usage.
Compatibility Testing
Ensure your plugin is compatible with:
- Popular themes.
- Other plugins.
- Different WordPress versions.
Debugging Tools
Log errors and debug issues with tools like Query Monitor and error logging in wp-config.php
:
define('WP_DEBUG', true);
define('WP_DEBUG_LOG', true);
Best Practices for Client-Specific Plugin Development
Follow these best practices for reliable and maintainable plugins:
- Use Descriptive Names: Name functions, classes, and files uniquely to avoid conflicts.
- Document Your Code: Provide comments and documentation to guide future developers.
- Ensure Security: Validate inputs, escape outputs, and follow WordPress security standards.
- Update Regularly: Maintain compatibility with WordPress updates and evolving client needs.
Conclusion
Client-specific plugin development is about creating tailored solutions that address unique challenges while aligning with business goals. By following best practices, optimizing for performance, and ensuring robust functionality, you can deliver plugins that exceed client expectations.
Start your development journey today and explore resources like the WordPress Plugin Handbook or WPBeginner’s Plugin Development Tutorials. With the right approach, you can build bespoke plugins that deliver exceptional results.