Testing WordPress plugins is a crucial step in ensuring that they function as intended, are free from bugs, and provide a seamless user experience. A thoroughly tested plugin not only improves reliability but also enhances compatibility with different WordPress themes, plugins, and versions. This guide explores best practices and tools for testing WordPress plugins, helping developers deliver high-quality products.
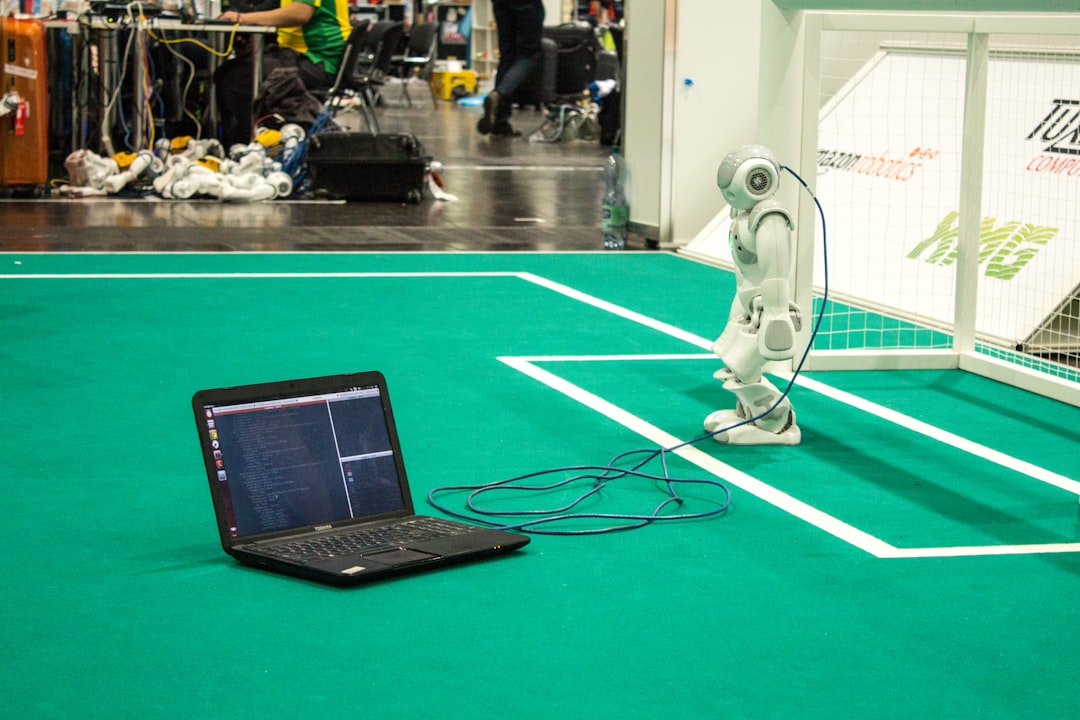
Why Testing WordPress Plugins is Essential
Testing is an integral part of the plugin development process. Here’s why focusing on testing WordPress plugins matters:
- Ensures Functionality: Detects bugs or errors in the plugin’s features before deployment.
- Improves Compatibility: Ensures the plugin works well with WordPress core updates, themes, and other plugins.
- Enhances Security: Identifies vulnerabilities to protect websites from potential threats.
- Boosts User Confidence: A well-tested plugin builds trust among users, leading to better reviews and adoption.
By investing in rigorous testing, developers can ensure their plugins perform reliably in real-world scenarios.
Best Practices for Testing WordPress Plugins
Testing plugins involves evaluating functionality, compatibility, performance, and security. Following best practices ensures thorough and efficient testing.
Test in a Staging Environment
Always test plugins in a staging environment to avoid affecting live websites. Tools like WP Staging create a replica of your site for safe testing.
Check Compatibility
Ensure your plugin is compatible with:
- Different versions of WordPress.
- Popular themes like Astra or GeneratePress.
- Widely used plugins like WooCommerce or Yoast SEO.
Test Across Devices and Browsers
Verify your plugin’s functionality on multiple devices (desktop, mobile, tablet) and browsers (Chrome, Firefox, Safari, Edge) to ensure a consistent user experience.
Validate Security Measures
Conduct penetration testing to identify vulnerabilities like SQL injection, cross-site scripting (XSS), or cross-site request forgery (CSRF). Use WordPress’s built-in security functions, such as sanitize_text_field()
and wp_verify_nonce()
.
Perform Load Testing
Evaluate your plugin’s performance under high-traffic conditions to ensure it can handle spikes in usage without slowing down the site.
For a detailed checklist, explore WordPress Plugin Developer Handbook.
Tools for Testing WordPress Plugins
A variety of tools are available to streamline and enhance the process of testing WordPress plugins. Here are some of the best options for different testing scenarios.
Unit Testing with PHPUnit
PHPUnit is the standard framework for testing PHP code. It’s ideal for testing individual functions and classes in your plugin.
Example: Setting up PHPUnit for WordPress:
- Install PHPUnit via Composer:
composer require --dev phpunit/phpunit
- Write a test case:
class PluginTest extends WP_UnitTestCase { public function test_example() { $this->assertTrue(true); } }
- Run tests:
vendor/bin/phpunit
Learn more about PHPUnit at PHPUnit Documentation.
Integration Testing with Codeception
Codeception extends PHPUnit, making it suitable for integration and functional testing. It supports browser-based testing with tools like Selenium.
Explore Codeception for WordPress.
Debugging with Query Monitor
Query Monitor is a powerful plugin for debugging performance, database queries, and PHP errors.
Features:
- Monitor slow database queries.
- Track hooks and actions.
- Debug REST API calls.
Download Query Monitor from WordPress.org.
Compatibility Testing with BrowserStack
BrowserStack allows you to test your plugin on real devices and browsers. It’s an excellent tool for cross-browser and cross-device compatibility.
Learn more at BrowserStack.
Performance Testing with New Relic
New Relic provides detailed performance analytics, helping you identify slow database queries, API calls, or other bottlenecks in your plugin.
Explore New Relic for WordPress.
Security Testing with WPScan
WPScan is a WordPress-specific vulnerability scanner that identifies security risks in plugins.
Features:
- Detect outdated plugins or themes.
- Identify insecure settings.
- Highlight vulnerabilities in code.
Discover more about WPScan at WPScan.
Load Testing with Apache JMeter
Apache JMeter helps simulate high traffic to evaluate how your plugin performs under stress.
Visit JMeter for more information.
Testing WordPress Plugins for Performance
Performance testing ensures your plugin doesn’t slow down the website or consume excessive resources.
Analyze Database Queries
Use Query Monitor to track database queries and optimize slow or redundant operations.
Example: Improving a database query:
$args = array(
'post_type' => 'custom_post',
'meta_key' => 'value',
'orderby' => 'meta_value',
'order' => 'DESC',
);
$query = new WP_Query($args);
Cache Expensive Operations
Use the Transients API to cache results of resource-intensive processes.
Example:
$data = get_transient('cached_data');
if (!$data) {
$data = fetch_expensive_data();
set_transient('cached_data', $data, HOUR_IN_SECONDS);
}
return $data;
Optimize Assets
Only load plugin assets on relevant pages to reduce unnecessary HTTP requests.
Example:
function load_plugin_assets($hook) {
if ('toplevel_page_plugin-settings' === $hook) {
wp_enqueue_style('plugin-style', plugin_dir_url(__FILE__) . 'css/style.css');
}
}
add_action('admin_enqueue_scripts', 'load_plugin_assets');
For performance optimization tips, visit Kinsta’s WordPress Performance Guide.
Debugging and Troubleshooting Plugins
Effective debugging ensures a smooth testing process and helps resolve issues quickly.
Enable WP_DEBUG
Activate WordPress debugging by adding the following to your wp-config.php
:
define('WP_DEBUG', true);
define('WP_DEBUG_LOG', true);
define('WP_DEBUG_DISPLAY', false);
Use Logging Tools
Log errors and events using the error_log()
function or dedicated plugins like Simple History.
Monitor REST API Responses
Debug API requests using tools like Query Monitor or Postman.
Handle Errors Gracefully
Always validate inputs and return descriptive error messages to help users troubleshoot issues.
Example:
if (!isset($_POST['nonce']) || !wp_verify_nonce($_POST['nonce'], 'plugin_nonce')) {
wp_send_json_error(array('message' => 'Invalid nonce'));
}
Conclusion
Testing WordPress plugins is a critical step in delivering reliable, secure, and high-performing products. By leveraging the best tools and adhering to thorough testing practices, you can ensure your plugin meets the highest standards of functionality and compatibility.
Start implementing these strategies for testing WordPress plugins today, and explore resources like the WordPress Plugin Handbook or WPBeginner’s Plugin Testing Tips. A well-tested plugin not only benefits your users but also builds your reputation as a reliable developer.