In today’s digital landscape, WordPress powers over 40% of websites, making it a leading platform for businesses and developers. While WordPress offers thousands of plugins to enhance functionality, some client needs require unique solutions. Developing custom WordPress plugins allows you to deliver tailored features and meet specific business goals. This guide explores the process, tools, and best practices for creating custom plugins that address client needs effectively.
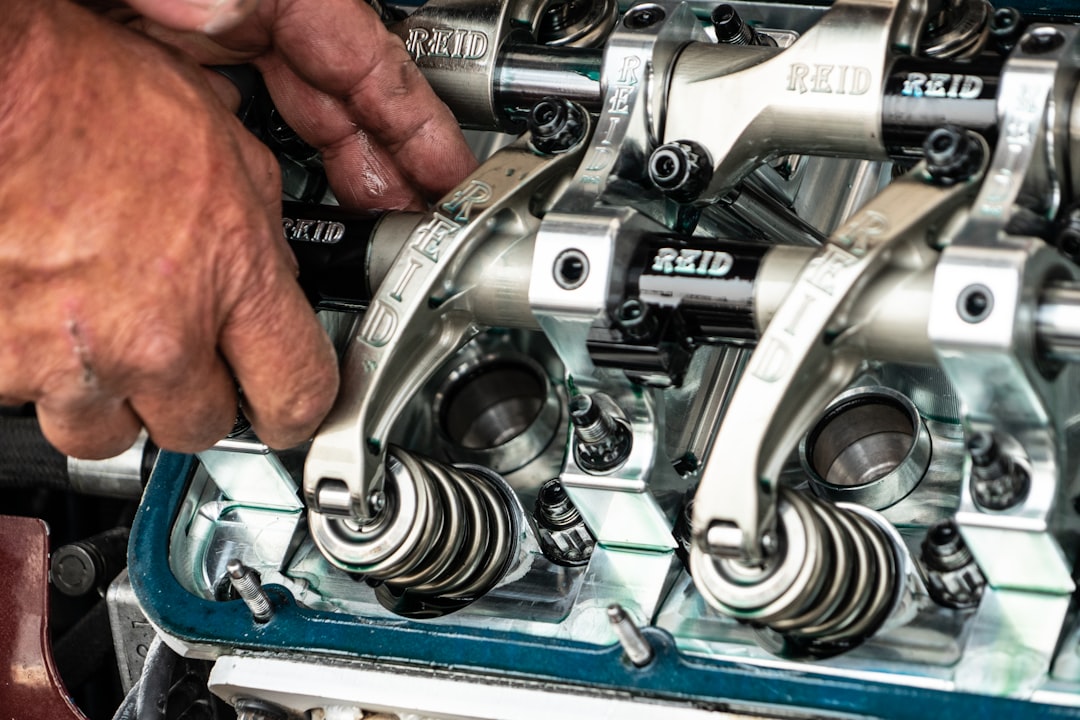
Why Choose Custom WordPress Plugins?
Off-the-shelf plugins often come with generic features, which may not align with a client’s exact requirements. Custom WordPress plugins solve this problem by providing tailored functionality, better integration with existing tools, and a cleaner user experience. They also enhance website performance by avoiding the bloat associated with overloading plugins.
For a deeper understanding of WordPress plugin development, explore the WordPress Plugin Handbook.
Understanding Client Requirements
Conduct a Needs Assessment
Start by understanding your client’s objectives. Identify the problem they want to solve or the functionality they need. Key questions to ask include:
- What is the primary goal of the plugin?
- What features should it include?
- How will it interact with the existing WordPress setup?
Define Use Cases
Translate client goals into clear use cases. For example, if your client runs an online store, a custom plugin might streamline inventory management or integrate with third-party logistics systems.
Prioritize Features
Work with your client to prioritize features. Focus on delivering core functionalities first, with options for adding advanced features later.
Setting Up Your Development Environment
Choose the Right Tools
For developing custom WordPress plugins, you’ll need the right tools. Recommended setups include:
- Code Editor: Use Visual Studio Code or PHPStorm for writing and debugging code.
- Local Development Environment: Set up a local WordPress environment using tools like Local by Flywheel or MAMP.
- Version Control: Use Git and GitHub to manage code changes and collaborate with teams.
For setup tutorials, visit Smashing Magazine’s guide.
Adhere to WordPress Standards
Follow WordPress coding standards to ensure compatibility and maintainability. Using these guidelines prevents errors and keeps your plugin aligned with best practices.
Building the Custom WordPress Plugin
Start with the Basics
Begin by creating a plugin folder and file within the WordPress directory. Add a header comment to define the plugin’s metadata:
<?php
/*
Plugin Name: Custom Plugin Example
Description: A custom plugin for specific client needs.
Version: 1.0
Author: Your Name
*/
Register Hooks and Actions
Use WordPress hooks and actions to integrate your plugin’s functionality seamlessly. Hooks like add_action()
and add_filter()
allow you to modify or add features without altering core WordPress files.
Example:
add_action('init', 'custom_plugin_init');
function custom_plugin_init() {
// Plugin initialization code
}
Build the Plugin Logic
Develop the core logic for your custom WordPress plugins based on client requirements. For instance, if the plugin manages user roles, write functions that add, edit, or delete roles as needed.
Ensure Compatibility
Test your plugin’s compatibility with different themes, WordPress versions, and other plugins. Use tools like Query Monitor to debug issues.
Optimizing Performance
Load Scripts and Styles Properly
To avoid performance issues, enqueue scripts and styles only when needed. Use wp_enqueue_script()
and wp_enqueue_style()
functions to load assets efficiently.
Example:
add_action('wp_enqueue_scripts', 'custom_plugin_scripts');
function custom_plugin_scripts() {
wp_enqueue_script('custom-plugin-script', plugin_dir_url(__FILE__) . 'js/custom-script.js', array('jquery'), '1.0', true);
}
Minimize Database Queries
Efficient database queries are essential for plugin performance. Use WordPress functions like $wpdb
for database interactions and avoid unnecessary queries.
Optimize Code
Write clean, modular code to improve readability and reduce bugs. Use PHP’s object-oriented programming (OOP) principles to organize your code into reusable components.
Adding Advanced Features
Create Admin Interfaces
Many custom WordPress plugins require an admin interface for settings or reports. Use the WordPress Settings API to create intuitive admin pages that allow clients to manage plugin configurations.
Example:
add_action('admin_menu', 'custom_plugin_menu');
function custom_plugin_menu() {
add_menu_page('Custom Plugin', 'Custom Plugin', 'manage_options', 'custom-plugin', 'custom_plugin_page');
}
function custom_plugin_page() {
echo '<h1>Custom Plugin Settings</h1>';
}
Implement Security Features
Security is critical in plugin development. Sanitize user inputs, validate data, and use nonces for form submissions to protect against common vulnerabilities.
Make the Plugin Multilingual
Support multiple languages by incorporating WordPress i18n functions like __()
and _e()
. Provide .po
files for translation to enhance usability for global clients.
Testing the Plugin
Perform Manual Testing
Test all features in a staging environment to ensure functionality. Check for compatibility issues with popular themes and plugins.
Use Automated Testing
Implement automated testing frameworks like PHPUnit for unit tests. Automated testing helps identify bugs early in the development cycle.
Get Client Feedback
Share the plugin with your client for testing and gather their feedback. Use their insights to refine the plugin and address specific concerns.
Delivering and Maintaining the Plugin
Provide Documentation
Comprehensive documentation is essential for client satisfaction. Include installation instructions, feature explanations, and troubleshooting tips.
Offer Ongoing Support
Custom WordPress plugins often require updates for bug fixes, security patches, and compatibility with newer WordPress versions. Set clear expectations with your client about maintenance and support.
Monitor Plugin Performance
Use analytics tools to monitor how the plugin performs on the client’s site. Track metrics like load times and usage patterns to identify areas for improvement.
For tips on ongoing support, explore Kinsta’s WordPress maintenance guide.
Advantages of Custom WordPress Plugins
Custom plugins offer unique benefits for both developers and clients. They enhance website functionality, improve user experience, and provide a competitive edge. By addressing specific needs, these plugins ensure that your client’s website stands out in a crowded market.
Conclusion
Building custom WordPress plugins is a rewarding process that combines technical skills with creativity. By understanding client requirements, adhering to best practices, and prioritizing performance, you can create plugins that deliver exceptional value. Whether it’s a simple feature enhancement or a complex functionality, custom plugins empower you to meet your client’s exact needs.
For additional resources, visit Codeable to connect with WordPress experts or the TutsPlus Plugin Development Hub for tutorials and insights. With dedication and expertise, custom plugin development can become a cornerstone of your WordPress services.