Creating robust and efficient WordPress plugins requires adherence to established coding standards. Following WordPress plugin standards ensures your code is clean, secure, and maintainable. It also helps maintain compatibility with WordPress core updates and other plugins. Whether you’re a seasoned developer or just starting with WordPress plugin development, adopting these standards is crucial to success.
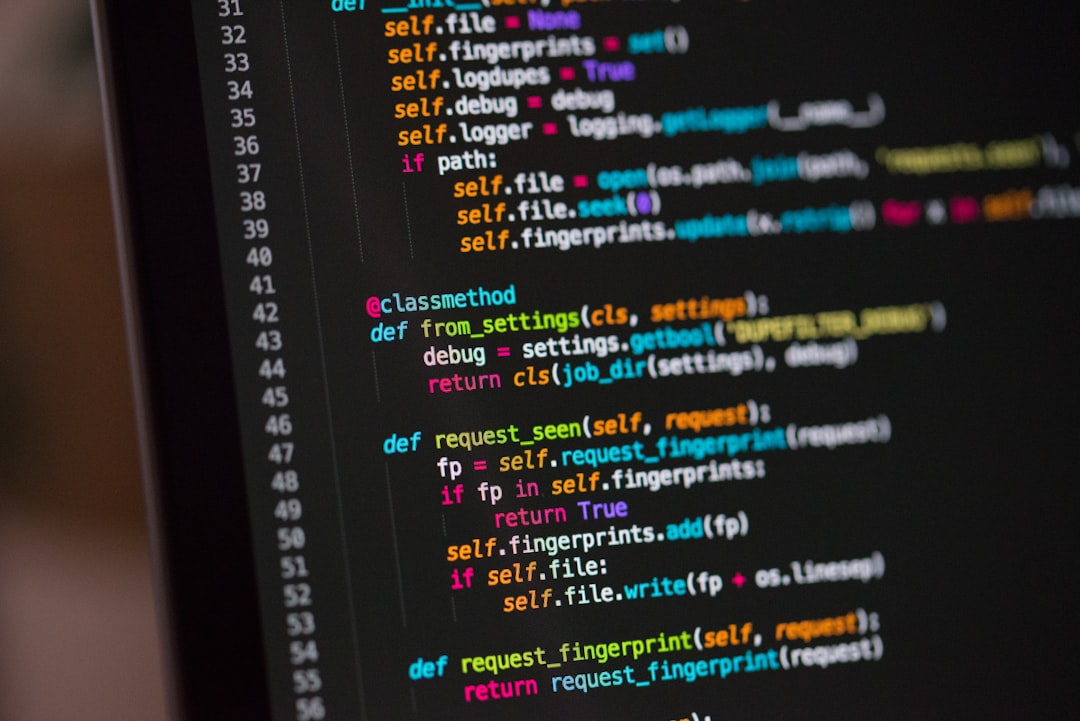
This guide outlines the essential coding guidelines for developing WordPress plugins, highlighting best practices and resources to streamline your workflow.
Why WordPress Plugin Standards Matter
WordPress plugin standards are not just a set of recommendations—they’re a necessity. They promote consistency, enhance collaboration among developers, and ensure your plugin functions correctly in diverse environments.
Benefits of following these standards include:
- Improved readability and maintainability.
- Enhanced security to protect users and websites.
- Compatibility with WordPress core, themes, and other plugins.
- Better chances of acceptance into the WordPress Plugin Repository.
For detailed documentation on coding standards, visit the WordPress Coding Standards Handbook.
Writing Clean and Readable Code
Follow PHP Coding Standards
WordPress plugins are primarily written in PHP, making it essential to follow the PHP coding standards outlined by WordPress. These standards include:
- Indentation using tabs, not spaces.
- Using meaningful variable names.
- Avoiding inline PHP in HTML files whenever possible.
Example:
function wp_plugin_example_function() {
$greeting = 'Hello, World!';
return $greeting;
}
For more details, explore the PHP Coding Standards.
Use Consistent Naming Conventions
Adopt consistent naming conventions for functions, variables, and classes to avoid conflicts with WordPress core or other plugins. Prefix all names with a unique identifier.
Example:
function myplugin_custom_function() {
// Custom code here
}
Organize Code with Comments
Comment your code to explain the purpose of functions and complex logic. Use DocBlocks to document functions, parameters, and return types.
Example:
/**
* Retrieves user data by ID.
*
* @param int $user_id The ID of the user.
* @return array $user_data The user data.
*/
function myplugin_get_user_data( $user_id ) {
// Fetch user data logic here
}
Ensuring Security in WordPress Plugins
Sanitize User Inputs
Always sanitize user inputs to prevent cross-site scripting (XSS) attacks. Use WordPress functions like sanitize_text_field()
and esc_url()
to clean data.
Example:
$sanitized_input = sanitize_text_field( $_POST['user_input'] );
Validate Data
Ensure all inputs meet expected formats or values before processing. Use conditional checks and WordPress validation functions like is_email()
or is_numeric()
.
Escape Outputs
Escape all data before outputting it to HTML to prevent XSS vulnerabilities. Use functions like esc_html()
or esc_attr()
.
Example:
echo esc_html( $user_data['name'] );
For more on plugin security, refer to OWASP Security Guidelines.
Using WordPress-Specific Functions
Use Built-In APIs
WordPress provides APIs to simplify plugin development while ensuring compatibility. Commonly used APIs include:
- Settings API: To create plugin settings pages.
- Shortcode API: To add custom shortcodes.
- REST API: To enable integration with external applications.
Example using the Settings API:
add_action( 'admin_init', 'myplugin_register_settings' );
function myplugin_register_settings() {
register_setting( 'myplugin_settings_group', 'myplugin_setting_name' );
}
Avoid Direct Database Queries
Direct database queries can be error-prone and compromise security. Instead, use $wpdb
, the WordPress Database API, to interact with the database.
Example:
global $wpdb;
$results = $wpdb->get_results( "SELECT * FROM {$wpdb->prefix}custom_table" );
Optimizing Plugin Performance
Load Scripts and Styles Correctly
Use wp_enqueue_script()
and wp_enqueue_style()
to load assets only when needed. This reduces unnecessary overhead.
Example:
add_action( 'wp_enqueue_scripts', 'myplugin_enqueue_assets' );
function myplugin_enqueue_assets() {
wp_enqueue_style( 'myplugin-style', plugin_dir_url( __FILE__ ) . 'css/style.css' );
wp_enqueue_script( 'myplugin-script', plugin_dir_url( __FILE__ ) . 'js/script.js', array( 'jquery' ), '1.0', true );
}
Optimize Database Queries
Minimize database queries by caching results and using transients for data that doesn’t change often.
Example:
$cached_data = get_transient( 'myplugin_data' );
if ( false === $cached_data ) {
// Fetch data
$cached_data = 'Fresh Data';
set_transient( 'myplugin_data', $cached_data, HOUR_IN_SECONDS );
}
Test Plugin Performance
Use tools like Query Monitor to identify and resolve performance bottlenecks. Track slow queries, memory usage, and asset loading times.
Ensuring Compatibility
Test with Popular Themes
Test your plugin with popular WordPress themes like Astra or GeneratePress to ensure it doesn’t cause layout or functionality issues.
Avoid Hardcoding Paths
Use WordPress functions like plugin_dir_path()
or plugins_url()
to generate paths dynamically. This ensures compatibility across environments.
Example:
include plugin_dir_path( __FILE__ ) . 'includes/admin-page.php';
Use Unique Prefixes
Prevent naming conflicts with themes or other plugins by prefixing functions, classes, and global variables.
Providing Documentation and Support
Write Comprehensive Documentation
Provide clear documentation for your plugin. Include installation steps, feature explanations, and examples of usage.
Add a Support System
Integrate a support system, such as a dedicated email address or a forum, to address user queries and resolve issues promptly.
Submitting to the WordPress Repository
Follow Repository Guidelines
Adhere to the WordPress Plugin Repository guidelines when submitting your plugin. These guidelines ensure quality, security, and compatibility.
Prepare a ReadMe File
Create a detailed readme.txt
file, including:
- Plugin description.
- Installation instructions.
- Changelog for updates.
For submission tips, explore Submitting Plugins to the WordPress Repository.
Conclusion
Adopting WordPress plugin standards is essential for developing secure, efficient, and user-friendly plugins. By following coding standards, prioritizing security, and optimizing performance, you can ensure your plugin stands out in the WordPress ecosystem.
For more guidance, visit resources like Codeable or TutsPlus to enhance your skills. Mastering these standards not only improves your plugins but also establishes your credibility as a professional WordPress developer.