Creating a WordPress plugin requires more than just adding functionality to a website. For a plugin to succeed, it must be well-coded, secure, and user-friendly. Adopting best practices for quality WordPress plugin development ensures your plugin performs efficiently and integrates seamlessly with the WordPress ecosystem.
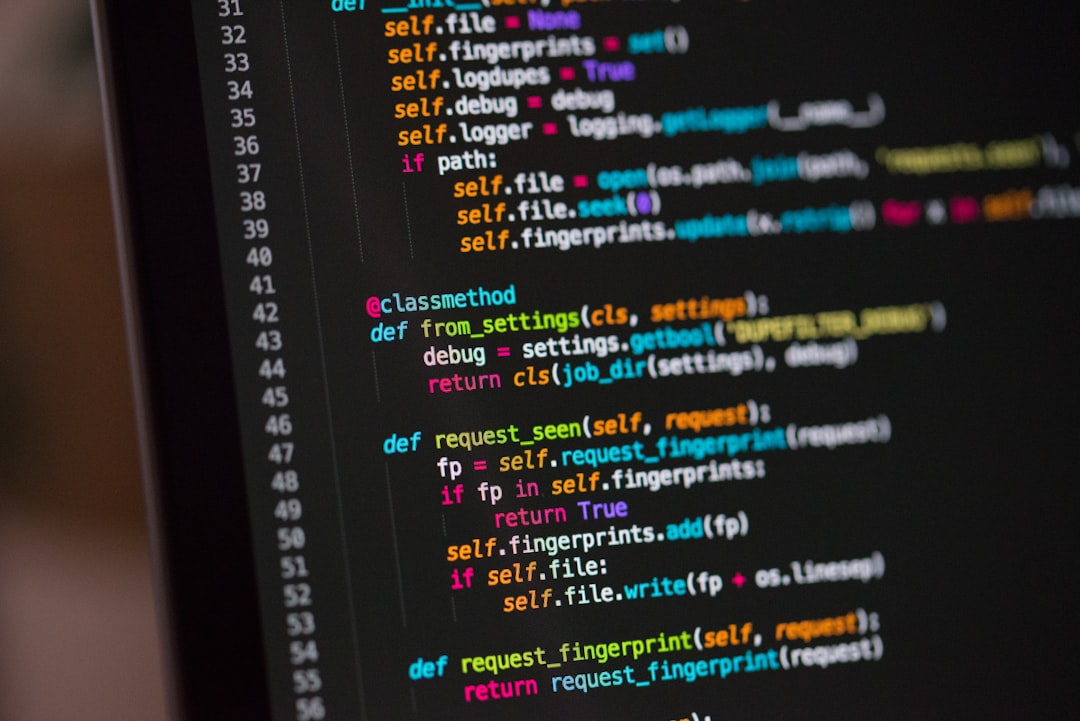
This guide covers the essential steps, tools, and strategies for building high-quality WordPress plugins that users and developers will trust and love.
Why Quality WordPress Plugin Development Matters
Plugins are integral to the WordPress experience, powering everything from simple widgets to complex applications. Poorly developed plugins can lead to:
- Security Risks: Vulnerabilities may expose websites to hacking.
- Performance Issues: Inefficient code can slow down websites.
- Compatibility Problems: Plugins must work with various themes and other plugins.
- Negative User Experience: Poor design or functionality leads to low adoption and bad reviews.
By focusing on quality WordPress plugin development, you can avoid these pitfalls and create reliable, effective plugins that meet user needs.
Setting Up Your Development Environment
Before writing code, establish a robust development environment to ensure efficiency and consistency.
Local Development Setup
Developing locally reduces errors and allows for easy testing. Use tools like:
- Local by Flywheel: A beginner-friendly local development environment.
- XAMPP or MAMP: Provides a local server to run WordPress.
- Docker: Offers a flexible container-based development setup.
Version Control with Git
Use Git for version control to track changes, collaborate with others, and rollback issues. Platforms like GitHub or GitLab integrate seamlessly with WordPress development.
Debugging Tools
WordPress debugging tools help identify and resolve issues during development. Enable debugging in wp-config.php
:
define('WP_DEBUG', true);
define('WP_DEBUG_LOG', true);
Plugins like Query Monitor are also invaluable for identifying performance bottlenecks and errors.
For more on setting up a development environment, visit the WordPress Developer Handbook.
Quality WordPress Plugin Development Best Practices
Adhering to coding standards and best practices ensures your plugin is efficient, secure, and maintainable.
Follow WordPress Coding Standards
WordPress coding standards ensure consistency and compatibility across plugins. Key guidelines include:
- Proper Indentation: Use spaces instead of tabs.
- Consistent Naming Conventions: Use snake_case for variables and functions.
- Readability: Write clear, well-commented code.
Example:
function my_plugin_custom_function() {
// Function logic here
}
Refer to the WordPress Coding Standards Guide for more details.
Use Hooks and Filters
Hooks and filters allow your plugin to integrate with WordPress without altering core files.
Example of an action hook:
add_action('wp_head', 'my_plugin_add_meta_tag');
function my_plugin_add_meta_tag() {
echo '<meta name="example" content="plugin">';
}
Using hooks ensures better compatibility with themes and other plugins.
Sanitize and Escape Data
Sanitizing and escaping data prevents vulnerabilities like SQL injection and cross-site scripting (XSS).
Example:
// Sanitize input
$input = sanitize_text_field($_POST['user_input']);
// Escape output
echo esc_html($input);
For more on security practices, see Sucuri’s Security Guide.
Quality WordPress Plugin Development for User Experience
A well-designed plugin provides a seamless and intuitive user experience.
Design an Intuitive Interface
Use WordPress’s native UI components to maintain a consistent look and feel. Libraries like the WordPress Settings API make it easier to create admin interfaces.
Example:
add_action('admin_menu', 'my_plugin_add_admin_page');
function my_plugin_add_admin_page() {
add_menu_page('My Plugin Settings', 'My Plugin', 'manage_options', 'my-plugin', 'my_plugin_settings_page');
}
function my_plugin_settings_page() {
echo '<h1>My Plugin Settings</h1>';
}
Optimize Performance
Avoid loading unnecessary scripts and styles. Use wp_enqueue_script
and wp_enqueue_style
to load assets conditionally.
Example:
add_action('wp_enqueue_scripts', 'my_plugin_load_assets');
function my_plugin_load_assets() {
if (is_page('specific-page')) {
wp_enqueue_script('my-plugin-script', plugin_dir_url(__FILE__) . 'js/script.js');
}
}
Test Across Devices and Browsers
Ensure your plugin works smoothly on different devices and browsers by using tools like BrowserStack or CrossBrowserTesting.
Testing and Debugging WordPress Plugins
Thorough testing ensures your plugin is reliable and bug-free before release.
Use Unit Testing
Automated tests validate your plugin’s functionality with minimal manual effort. Use the PHPUnit framework for testing WordPress plugins.
Test Compatibility
Check your plugin’s compatibility with various themes and plugins to prevent conflicts. Use a staging site to test real-world scenarios.
Monitor Performance
Analyze your plugin’s impact on website performance using tools like New Relic or Query Monitor. Optimize heavy database queries or resource-intensive operations.
Debug Errors
Enable error logging in WordPress to identify issues:
define('WP_DEBUG', true);
define('WP_DEBUG_LOG', true);
Review the debug.log
file in the wp-content
directory for error messages.
Maintaining and Updating Your Plugin
A plugin’s success depends on consistent maintenance and updates to meet user needs and adapt to WordPress core changes.
Respond to User Feedback
Monitor plugin reviews and support forums to identify user concerns and implement improvements.
Regularly Update Your Plugin
Keep your plugin compatible with the latest WordPress version and fix any security vulnerabilities.
Use Semantic Versioning
Adopt a clear versioning system to communicate updates. For example:
- Major Updates: Introduce significant changes (e.g., 2.0.0).
- Minor Updates: Add features or improvements (e.g., 2.1.0).
- Patch Updates: Fix bugs (e.g., 2.1.1).
Maintain Documentation
Provide clear and detailed documentation for users and developers. Include setup instructions, FAQs, and examples.
For tips on maintaining plugins, visit WPBeginner’s Plugin Guide.
Conclusion
Building a high-quality WordPress plugin requires careful planning, adherence to coding standards, and a focus on user experience. By implementing best practices for quality WordPress plugin development, you can create plugins that are secure, efficient, and user-friendly.
Start building your plugin with these strategies, and watch as it becomes a trusted tool for WordPress users. For more resources, explore the WordPress Developer Handbook or Kinsta’s Plugin Development Tips.