WooCommerce powers millions of online stores, offering flexibility and scalability to businesses of all sizes. However, store owners often need unique functionalities that go beyond the standard WooCommerce features. This is where custom plugins WooCommerce come into play. By developing custom plugins, developers can tailor online stores to meet specific business requirements, enhance user experience, and streamline operations.
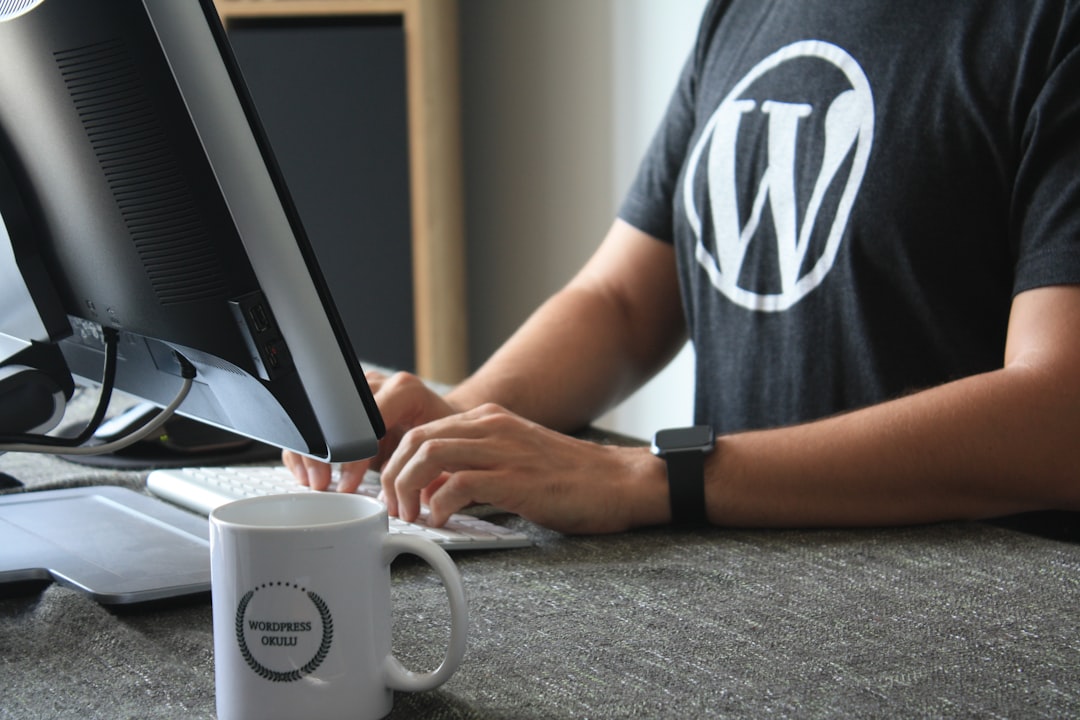
This guide explores the steps, best practices, and tools for developing custom plugins for WooCommerce stores.
Why Develop Custom Plugins WooCommerce?
WooCommerce provides extensive functionality, but no plugin can address every possible business scenario. Custom plugins allow you to:
- Add unique features tailored to your store’s needs.
- Automate specific tasks to save time and improve efficiency.
- Integrate third-party services or APIs for advanced capabilities.
- Enhance the overall user experience by addressing specific pain points.
For more on WooCommerce customization, visit WooCommerce Developer Resources.
Setting Up Your Development Environment
Install WordPress and WooCommerce Locally
Before building custom plugins WooCommerce, set up a local development environment. Tools like Local by Flywheel or XAMPP simplify the process, allowing you to test your plugins safely.
Steps:
- Install WordPress locally.
- Download and activate WooCommerce.
- Create sample products and categories for testing.
Choose a Code Editor
Use a code editor like Visual Studio Code or PHPStorm for writing plugin code. These editors offer features like syntax highlighting, debugging, and extension support.
Explore Visual Studio Code for a free, developer-friendly editor.
Set Up Version Control
Implement version control with Git to track changes and collaborate effectively. Platforms like GitHub or Bitbucket can host your repositories for easy access.
Creating a Custom Plugin for WooCommerce
Structure Your Plugin
Organize your plugin files to follow WordPress and WooCommerce conventions. A standard structure includes:
/my-custom-plugin/
/includes/
/assets/
main-plugin-file.php
Add the Plugin Header
The main plugin file should include a header comment with metadata to identify your plugin.
<?php
/*
Plugin Name: Custom WooCommerce Plugin
Description: Adds custom functionality to WooCommerce.
Version: 1.0
Author: Your Name
*/
Hook into WooCommerce
WooCommerce offers hooks and filters to modify its behavior without altering the core files. Use these to add or customize functionality.
Example: Adding a custom message to the cart page.
add_action( 'woocommerce_before_cart', 'custom_cart_message' );
function custom_cart_message() {
echo '<p>Thank you for shopping with us!</p>';
}
Adding Functionality with Custom Plugins WooCommerce
Create Custom Product Types
WooCommerce supports custom product types, such as subscriptions or bookings. Use the WC_Product
class to define new product types.
Example: Creating a “Gift Card” product type.
class WC_Product_Gift_Card extends WC_Product {
public function __construct( $product ) {
$this->product_type = 'gift_card';
parent::__construct( $product );
}
}
Register the product type:
add_filter( 'product_type_selector', 'add_gift_card_product_type' );
function add_gift_card_product_type( $types ) {
$types['gift_card'] = __( 'Gift Card', 'textdomain' );
return $types;
}
Customize Checkout Fields
Modify the checkout experience by adding or removing fields. Use the woocommerce_checkout_fields
filter to manage these fields.
Example: Adding a “Company Name” field:
add_filter( 'woocommerce_checkout_fields', 'add_company_name_field' );
function add_company_name_field( $fields ) {
$fields['billing']['billing_company_name'] = array(
'type' => 'text',
'label' => __( 'Company Name', 'textdomain' ),
'required' => false,
);
return $fields;
}
Automate Tasks
Custom plugins WooCommerce can automate tasks such as inventory updates, email notifications, or reporting. Use WooCommerce’s action hooks to trigger these tasks.
Example: Sending a custom email when a product’s stock is low.
add_action( 'woocommerce_low_stock', 'notify_admin_low_stock' );
function notify_admin_low_stock( $product ) {
$to = get_option( 'admin_email' );
$subject = 'Low Stock Alert';
$message = 'The product ' . $product->get_name() . ' is running low on stock.';
wp_mail( $to, $subject, $message );
}
Optimizing Plugin Performance
Load Assets Conditionally
Only load scripts and styles on WooCommerce pages to improve performance.
add_action( 'wp_enqueue_scripts', 'load_custom_plugin_assets' );
function load_custom_plugin_assets() {
if ( is_woocommerce() ) {
wp_enqueue_style( 'custom-plugin-style', plugin_dir_url( __FILE__ ) . 'assets/style.css' );
}
}
Minimize Database Queries
Use transients or caching to store data and reduce database queries.
$cached_data = get_transient( 'custom_plugin_data' );
if ( false === $cached_data ) {
$cached_data = expensive_query_function();
set_transient( 'custom_plugin_data', $cached_data, 12 * HOUR_IN_SECONDS );
}
Ensuring Compatibility
Test with WooCommerce Themes
Test your custom plugin with popular WooCommerce themes like Astra or Storefront to identify and fix compatibility issues.
Follow WooCommerce Coding Standards
Adhere to the WooCommerce coding standards to ensure your plugin integrates seamlessly. Visit the WooCommerce GitHub Repository for coding guidelines.
Providing Documentation and Support
Create a User Guide
Include a detailed user guide with installation instructions, feature explanations, and troubleshooting tips.
Offer Support Channels
Provide email or ticket-based support to address user queries and resolve issues. Tools like Zendesk can streamline this process.
Collect Feedback
Encourage users to provide feedback on your custom plugin. Use this input to improve functionality and user experience.
Deploying Custom Plugins WooCommerce
Package Your Plugin
Zip your plugin files, ensuring all necessary components are included. The structure should look like this:
/my-custom-plugin.zip
/includes/
/assets/
main-plugin-file.php
Test in a Staging Environment
Before deploying, test your plugin on a staging site to ensure it functions correctly under real-world conditions.
Publish and Update
Publish your plugin on a private repository or distribute it to clients directly. Provide regular updates to maintain compatibility with WooCommerce core.
Conclusion
Developing custom plugins WooCommerce allows you to create tailored solutions that meet unique store requirements. By understanding WooCommerce’s hooks, filters, and APIs, you can add advanced features, automate tasks, and optimize the user experience.
A strong local development environment, adherence to coding standards, and a focus on performance ensure your plugins are effective and reliable. For further learning, visit Codeable or the TutsPlus WooCommerce Development Guide. Custom plugin development empowers developers to deliver unparalleled value to WooCommerce stores.