WordPress plugins add tremendous functionality and flexibility to websites, but they can also introduce security vulnerabilities if not developed or maintained properly. Hackers frequently target plugins to exploit weaknesses, gain unauthorized access, or disrupt operations. Understanding WordPress plugin security vulnerabilities and implementing strategies to mitigate them is critical for protecting your website.
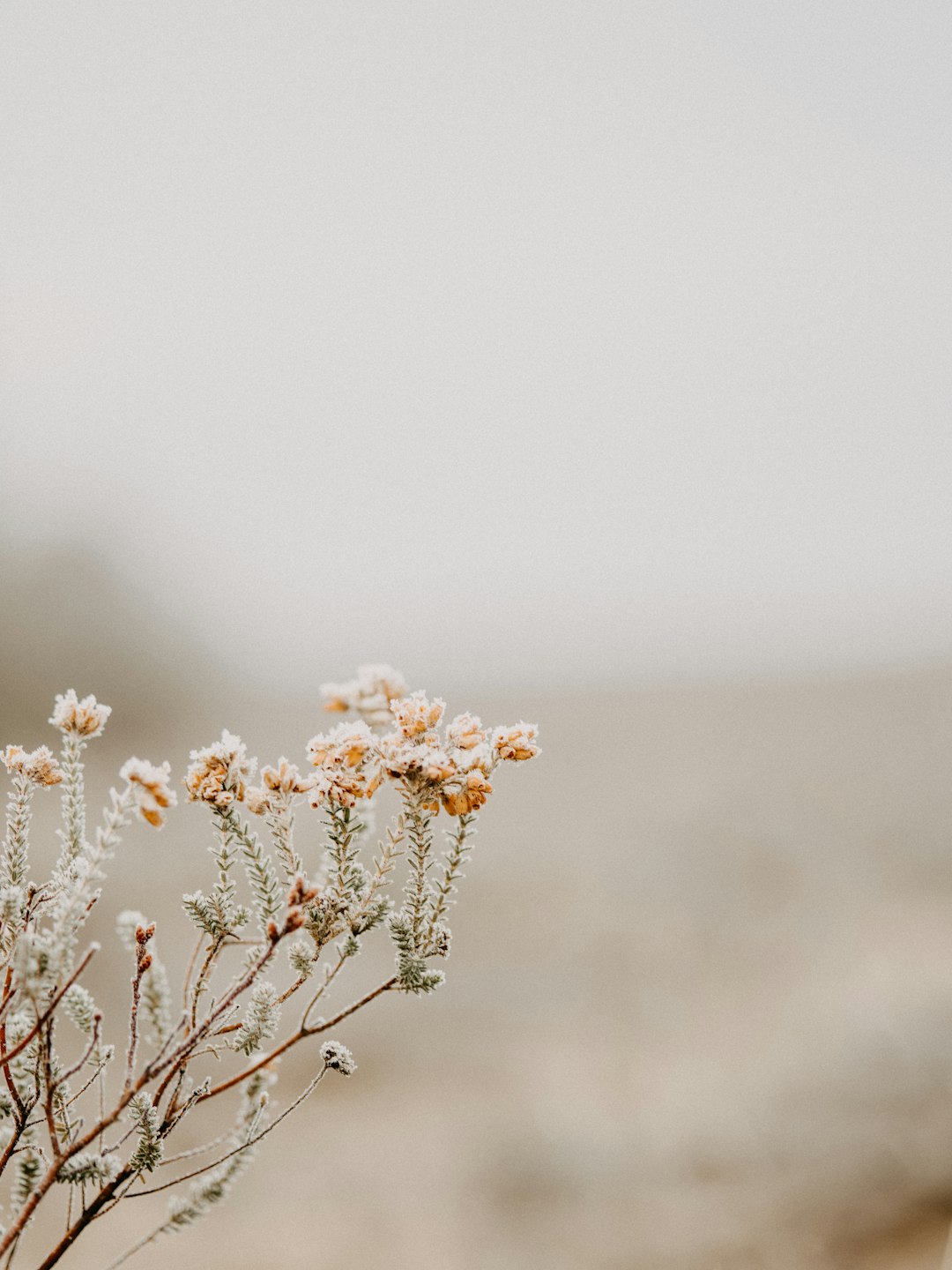
This guide explores common security flaws, real-world examples, and actionable steps to develop and manage plugins safely.
Common WordPress Plugin Security Vulnerabilities
Plugins interact directly with your WordPress site’s database, files, and user data. If improperly coded or configured, they can become entry points for attackers. Below are the most common vulnerabilities:
SQL Injection
SQL injection occurs when attackers manipulate database queries to gain access or control over data. This often results from unvalidated or unsanitized user input in plugin forms or APIs.
Cross-Site Scripting (XSS)
XSS vulnerabilities allow hackers to inject malicious scripts into your site’s front end, potentially stealing user credentials or spreading malware.
Cross-Site Request Forgery (CSRF)
CSRF attacks trick authenticated users into performing actions they didn’t intend, such as changing passwords or transferring funds, by exploiting their active sessions.
File Inclusion Vulnerabilities
Improper handling of file paths can enable attackers to include malicious files, potentially compromising the entire website.
Weak Authentication
Plugins that fail to enforce secure authentication practices, like strong password policies or multi-factor authentication, increase the risk of unauthorized access.
For a deeper understanding of these vulnerabilities, visit OWASP’s Top 10 Security Risks.
SQL Injection in WordPress Plugin Security Vulnerabilities
SQL injection remains one of the most significant threats to WordPress plugins, allowing attackers to manipulate or destroy data.
How SQL Injection Happens
SQL injection occurs when unsanitized user input is used directly in database queries. For example:
$input = $_GET['id'];
$result = $wpdb->get_results("SELECT * FROM wp_table WHERE id = $input");
In this case, an attacker could manipulate the $input
variable to execute malicious SQL commands.
How to Prevent SQL Injection
Using prepared statements in WordPress’s $wpdb
class protects your queries.
Example:
$input = intval($_GET['id']);
$result = $wpdb->get_results($wpdb->prepare("SELECT * FROM wp_table WHERE id = %d", $input));
This approach ensures user input is properly sanitized before being passed to the database.
For additional tips, explore WordPress SQL Injection Prevention.
Cross-Site Scripting in WordPress Plugin Security Vulnerabilities
XSS vulnerabilities allow attackers to inject harmful scripts into your site, often targeting unsuspecting users.
How XSS Happens
XSS attacks commonly occur when a plugin displays unsanitized user input directly on the website. For example:
echo $_GET['name'];
If an attacker provides malicious input, the script executes in the browser, potentially stealing cookies or session data.
How to Prevent XSS
Always sanitize and escape user input before displaying it. WordPress provides several built-in functions for this purpose:
esc_html()
: Escapes HTML output.esc_url()
: Sanitizes URLs.esc_attr()
: Escapes attribute values.
Example:
$name = sanitize_text_field($_GET['name']);
echo esc_html($name);
For more guidance, check out the WordPress XSS Prevention Guide.
Mitigating Other WordPress Plugin Security Vulnerabilities
Beyond SQL injection and XSS, several other vulnerabilities require attention to maintain plugin security.
Preventing CSRF Attacks
CSRF attacks exploit trust in authenticated users to perform unauthorized actions. To mitigate this, use nonces to validate requests.
Example:
wp_nonce_field('plugin_action', 'plugin_nonce');
if (!isset($_POST['plugin_nonce']) || !wp_verify_nonce($_POST['plugin_nonce'], 'plugin_action')) {
die('Security check failed');
}
This ensures only legitimate requests are processed.
Securing File Uploads
Plugins that allow file uploads must validate file types and restrict executable file formats.
Example:
$allowed_types = array('jpg', 'png', 'pdf');
$file_type = wp_check_filetype($_FILES['uploaded_file']['name']);
if (!in_array($file_type['ext'], $allowed_types)) {
die('Invalid file type');
}
Using Secure Authentication
Implement strong password requirements and support multi-factor authentication to improve user account security.
For additional practices, explore Sucuri’s Security Blog.
Managing Updates and Third-Party Integrations
Keeping plugins updated and managing third-party integrations are vital to minimizing vulnerabilities.
Regularly Update Plugins
Outdated plugins often contain vulnerabilities that hackers can exploit. Regular updates patch these flaws and improve compatibility with WordPress core updates.
Vet Third-Party Code
Using third-party libraries can introduce risks if they are poorly maintained or outdated. Always use reputable sources and verify compatibility with your plugin.
Monitor Vulnerability Databases
Stay informed about known plugin vulnerabilities by subscribing to resources like WPScan or NVD (National Vulnerability Database).
Testing and Debugging for Security
Testing and debugging are essential for identifying and resolving vulnerabilities during development.
Use Staging Environments
Always test plugins in a staging environment before deploying to production. This minimizes the risk of affecting live sites.
Conduct Penetration Testing
Simulate attacks to identify potential vulnerabilities in your plugin. Tools like Burp Suite and OWASP ZAP are excellent for penetration testing.
Log and Monitor Activity
Implement logging mechanisms to monitor user and plugin activity for suspicious behavior. Use plugins like Activity Log to track changes.
Best Practices for Securing WordPress Plugins
Adhering to best practices during development and maintenance ensures a secure plugin ecosystem:
- Follow Coding Standards: Adhere to the official WordPress Coding Standards.
- Limit Permissions: Restrict access to sensitive files and directories to minimize exposure.
- Implement HTTPS: Ensure all data transmitted between users and your site is encrypted using HTTPS.
- Conduct Code Reviews: Regularly audit your codebase for potential security issues.
Conclusion
Understanding and addressing WordPress plugin security vulnerabilities is crucial for building reliable, secure plugins that users trust. By following secure coding practices, staying updated, and testing thoroughly, you can protect your plugins and the websites that rely on them.
Start implementing these strategies today, and explore additional resources like the WordPress Plugin Developer Handbook or Kinsta’s Security Guide.