WordPress plugins are essential for adding functionality and enhancing user experience, but they can also impact website performance if not optimized correctly. Slow-loading plugins can lead to higher bounce rates, poor user experiences, and lower search engine rankings. Understanding the importance of optimizing WordPress plugins is crucial for maintaining a fast and reliable website.
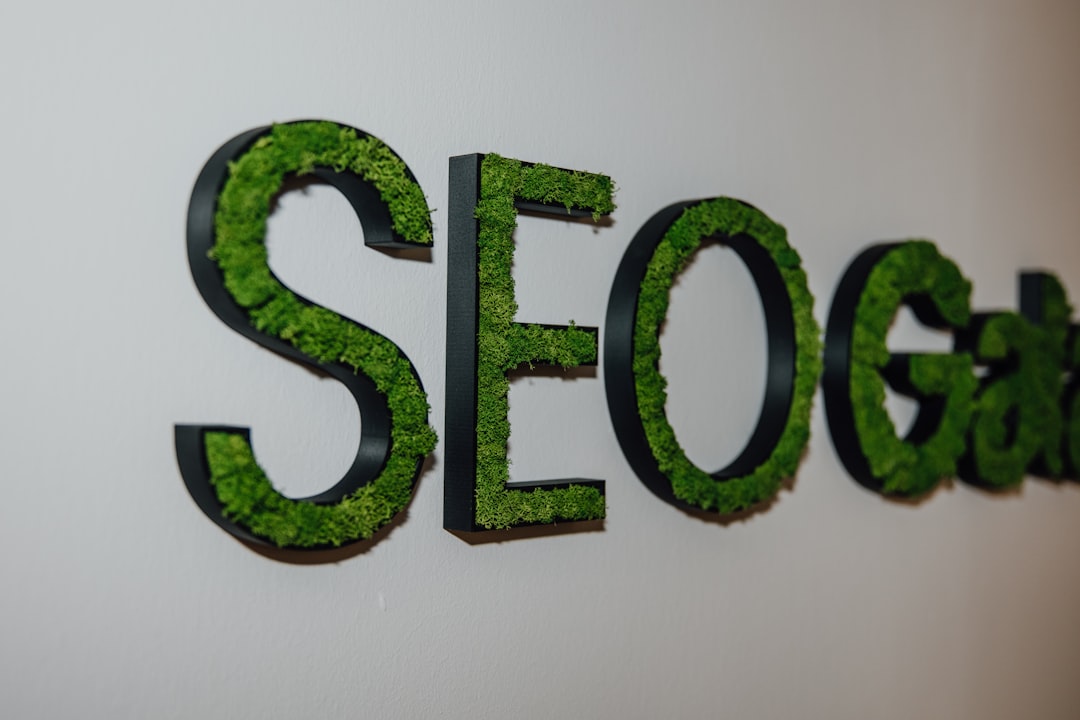
This guide explores best practices for plugin optimization, common pitfalls to avoid, and actionable strategies to improve plugin performance without sacrificing functionality.
Why Optimizing WordPress Plugins Matters
Plugins interact with your WordPress site’s code, database, and front-end resources. When poorly optimized, they can cause:
- Longer Load Times: Resource-intensive plugins can delay page rendering.
- Increased Server Load: Excessive database queries and scripts can strain hosting resources.
- SEO Challenges: Search engines prioritize fast-loading websites, so slow plugins can harm rankings.
By focusing on optimizing WordPress plugins, you ensure better user experiences, improved search engine visibility, and enhanced site stability.
Identifying Performance Bottlenecks
The first step in plugin optimization is identifying the source of performance issues.
Use Performance Monitoring Tools
Several tools can help you identify plugin-related performance bottlenecks:
- Query Monitor: Highlights slow database queries and plugin-related errors.
- GTmetrix: Analyzes page load times and identifies plugins causing delays.
- New Relic: Monitors server-side performance and pinpoints resource-heavy plugins.
Analyze Database Impact
Some plugins execute frequent or inefficient queries, leading to slower performance. Use tools like phpMyAdmin or WP-Optimize to review query logs and pinpoint problematic plugins.
For detailed performance insights, explore Kinsta’s Guide to WordPress Performance Monitoring.
Optimizing WordPress Plugins Through Code Efficiency
Efficient coding practices are key to optimizing plugin performance.
Reduce HTTP Requests
Plugins often load additional JavaScript or CSS files, increasing the number of HTTP requests. Combining and minifying these files reduces page load times.
Example using wp_enqueue_script
:
function optimize_plugin_assets() {
wp_enqueue_script('plugin-script', plugin_dir_url(__FILE__) . 'js/script.js', array('jquery'), null, true);
wp_enqueue_style('plugin-style', plugin_dir_url(__FILE__) . 'css/style.css');
}
add_action('wp_enqueue_scripts', 'optimize_plugin_assets');
To combine and minify assets, consider using plugins like Autoptimize.
Optimize Database Queries
Ensure database queries are efficient and use indexes where necessary.
Example of a prepared query:
global $wpdb;
$results = $wpdb->get_results($wpdb->prepare("SELECT * FROM wp_table WHERE column = %s", $value));
Prepared statements protect against SQL injection while improving query performance.
Avoid Overusing Hooks and Filters
While hooks and filters are powerful, excessive or redundant usage can slow down your site. Audit your plugin’s code to ensure only necessary hooks are implemented.
For more tips, visit WordPress Coding Standards.
Optimizing WordPress Plugins with Caching
Caching can significantly reduce the load times of resource-intensive plugins.
Use Object Caching
Object caching stores the results of database queries in memory, reducing the need for repeated queries. Plugins like Redis Object Cache integrate seamlessly with WordPress.
Implement Transients API
The Transients API allows you to store temporary data in the database for better performance.
Example:
$data = get_transient('plugin_cache_data');
if (!$data) {
$data = fetch_external_data();
set_transient('plugin_cache_data', $data, HOUR_IN_SECONDS);
}
Leverage Page Caching
Use caching plugins like WP Rocket to store static versions of your pages and bypass plugin-heavy processing.
Explore more caching techniques on WPBeginner’s Caching Guide.
Optimizing WordPress Plugins for Front-End Performance
Front-end optimization ensures plugins don’t unnecessarily impact your website’s loading speed.
Load Scripts Conditionally
Avoid loading scripts on pages where they aren’t needed.
Example:
function conditional_plugin_scripts() {
if (is_page('specific-page')) {
wp_enqueue_script('plugin-specific-script', plugin_dir_url(__FILE__) . 'js/script.js');
}
}
add_action('wp_enqueue_scripts', 'conditional_plugin_scripts');
Lazy Load Non-Essential Features
Lazy loading delays the loading of non-critical elements until they are needed. This improves initial load times.
Defer or Async JavaScript
Loading JavaScript asynchronously or deferring its execution prevents it from blocking page rendering.
Example using the script_loader_tag
filter:
add_filter('script_loader_tag', function($tag, $handle) {
if ('plugin-script-handle' === $handle) {
return str_replace('src', 'async="async" src', $tag);
}
return $tag;
}, 10, 2);
For front-end optimization strategies, explore Google’s PageSpeed Insights.
Managing Active Plugins
Managing the number of active plugins and their impact on your website is a critical aspect of optimization.
Deactivate and Delete Unused Plugins
Inactive plugins can still load autoloaded data, affecting performance. Remove any plugins that are no longer in use.
Consolidate Plugin Functionality
If multiple plugins perform similar tasks, consolidate their functionality into a single, lightweight plugin.
Choose Lightweight Alternatives
Opt for plugins with a smaller codebase and fewer dependencies. For example, use Rank Math for SEO instead of multiple SEO tools.
For plugin management tips, check out WPBeginner’s Plugin Tips.
Testing and Debugging Plugin Performance
Regular testing ensures that plugin optimization efforts are effective and sustainable.
Use Debugging Tools
Tools like Query Monitor and New Relic can help debug slow plugins and identify performance bottlenecks.
Test in Staging Environments
Always test plugin changes in a staging environment before deploying them to your live site. Use staging tools like WP Staging.
Monitor Performance Over Time
Regularly monitor your site’s performance using tools like GTmetrix or Pingdom Tools.
Conclusion
Optimizing WordPress plugins is essential for improving website performance, enhancing user experience, and maintaining SEO rankings. By focusing on efficient coding, caching strategies, and front-end optimizations, you can ensure your plugins contribute positively to your site’s performance.
Start implementing these strategies today to keep your WordPress site running smoothly. For additional resources, explore Kinsta’s Performance Blog or WP Rocket’s Optimization Tips.